


Best Practices for Secure File Uploads in PHP: Preventing Common Vulnerabilities
How to Handle File Uploads Securely in PHP
File uploads are a common feature in web applications, allowing users to share files like images, documents, or videos. However, file uploads come with security risks if not handled properly. Improperly handled uploads can lead to vulnerabilities such as remote code execution, overwriting critical files, and denial of service attacks.
To mitigate these risks, it’s essential to implement secure practices when handling file uploads in PHP. Below is a comprehensive guide on securely handling file uploads in PHP, covering best practices, common vulnerabilities, and techniques to secure file uploads.
1. Basic File Upload in PHP
In PHP, file uploads are handled through the $_FILES superglobal, which stores information about the uploaded files. Here's a basic example of how file uploads work:
// HTML form for file upload
The above is the detailed content of Best Practices for Secure File Uploads in PHP: Preventing Common Vulnerabilities. For more information, please follow other related articles on the PHP Chinese website!
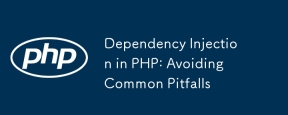
DependencyInjection(DI)inPHPenhancescodeflexibilityandtestabilitybydecouplingdependencycreationfromusage.ToimplementDIeffectively:1)UseDIcontainersjudiciouslytoavoidover-engineering.2)Avoidconstructoroverloadbylimitingdependenciestothreeorfour.3)Adhe
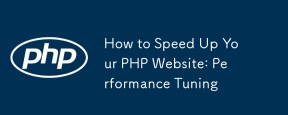
ToimproveyourPHPwebsite'sperformance,usethesestrategies:1)ImplementopcodecachingwithOPcachetospeedupscriptinterpretation.2)Optimizedatabasequeriesbyselectingonlynecessaryfields.3)UsecachingsystemslikeRedisorMemcachedtoreducedatabaseload.4)Applyasynch
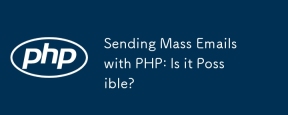
Yes,itispossibletosendmassemailswithPHP.1)UselibrarieslikePHPMailerorSwiftMailerforefficientemailsending.2)Implementdelaysbetweenemailstoavoidspamflags.3)Personalizeemailsusingdynamiccontenttoimproveengagement.4)UsequeuesystemslikeRabbitMQorRedisforb
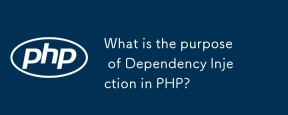
DependencyInjection(DI)inPHPisadesignpatternthatachievesInversionofControl(IoC)byallowingdependenciestobeinjectedintoclasses,enhancingmodularity,testability,andflexibility.DIdecouplesclassesfromspecificimplementations,makingcodemoremanageableandadapt
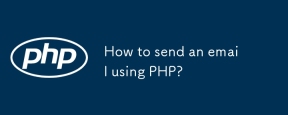
The best ways to send emails using PHP include: 1. Use PHP's mail() function to basic sending; 2. Use PHPMailer library to send more complex HTML mail; 3. Use transactional mail services such as SendGrid to improve reliability and analysis capabilities. With these methods, you can ensure that emails not only reach the inbox, but also attract recipients.
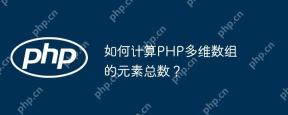
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
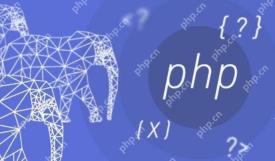
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
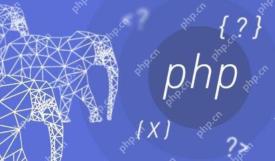
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
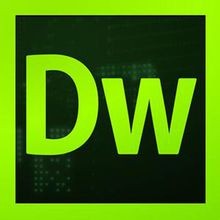
Dreamweaver CS6
Visual web development tools
