Setting Property Values Using Reflection
It is possible to dynamically set the value of a property using reflection in C#. This allows you to modify an object's property at runtime, regardless of its accessibility or visibility.
To set a property value using reflection, follow these steps:
- Get the PropertyInfo Object: Use Type.GetProperty to retrieve the PropertyInfo object associated with the property you want to modify. If the property is not public, you may need to specify additional binding flags, such as BindingFlags.NonPublic or BindingFlags.Instance.
- Invoke the SetValue Method: Once you have the PropertyInfo object, invoke its SetValue method to actually set the value of the property. This method takes two parameters: the object instance you want to modify and the new value to set.
Here's an example that demonstrates how to use reflection to set the firstName property of a Person class:
using System; using System.Reflection; class Person { public string FirstName { get; set; } } class Test { static void Main(string[] args) { // Create an instance of the Person class Person p = new Person(); // Get the PropertyInfo object for the FirstName property var property = typeof(Person).GetProperty("FirstName"); // Set the value of the FirstName property using reflection property.SetValue(p, "John", null); // Print the value of the FirstName property Console.WriteLine(p.FirstName); // John } }
In this example, the property variable holds a reference to the FirstName property of the Person class. The SetValue method is invoked with the p instance and the string value "John" to set the property's value dynamically.
The above is the detailed content of How Can I Set Property Values Dynamically in C# Using Reflection?. For more information, please follow other related articles on the PHP Chinese website!
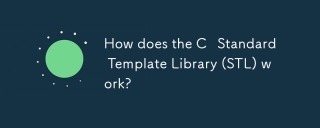
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
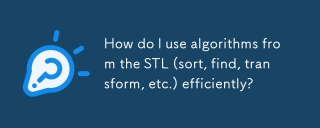
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
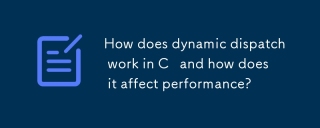
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
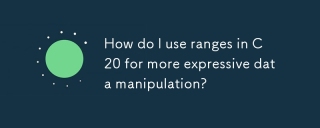
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
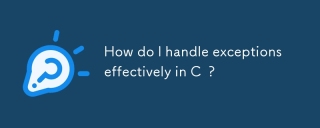
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
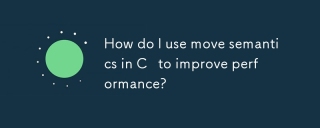
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
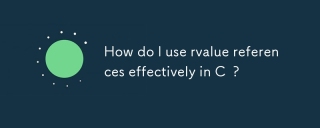
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
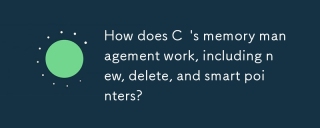
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
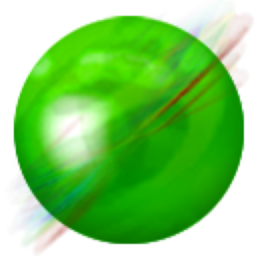
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
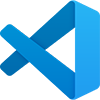
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
