


Understanding Python's any() and all() Functions
Built-in functions in Python, any() and all(), play a crucial role in evaluating the truthiness of values within an iterable. These functions offer concise and efficient ways of testing multiple conditions.
any() Function
any() determines whether any element in an iterable is True. It returns True if even a single True value is present; otherwise, it returns False.
Example:
my_list = [0, False, 'Hello', 1, ''] print(any(my_list)) # Outputs True as 'Hello' and 1 are True
all() Function
all(), on the other hand, checks if every element in an iterable is True. It only returns True if all elements are True; otherwise, it returns False.
Example:
my_list = [True, 1, 'True'] print(all(my_list)) # Outputs True as all elements are True
Truthiness and Logical Evaluation
any() and all() essentially perform logical OR and AND operations, respectively. Understanding their truthiness behavior is essential.
Truth Table:
Iterable Values | any() | all() |
---|---|---|
All True | True | True |
All False | False | False |
Mixed True and False | True | False |
Empty Iterable | False | True |
Understanding Your Code
In your code, you utilize a combination of any() and all() to check if any value in a tuple is different while ensuring that not all values are different. However, the output you expected was not obtained because of a misunderstanding in the code's evaluation:
d = defaultdict(list) print(list(zip(*d['Drd2']))) # [(1, 1), (5, 6), (0, 0)] print([any(x) and not all(x) for x in zip(*d['Drd2'])]) # [False, False, False]
In this case, any(x) checks if any value in a tuple is different (i.e., True), while all(x) ensures that not all values are different (i.e., False). Since (1, 1), (5, 6), and (0, 0) all have different values, it correctly evaluates all three tuples as [False, False, False].
To achieve your desired output, you could modify the code as follows:
print([x[0] != x[1] for x in zip(*d['Drd2'])]) # [False, True, False]
This directly checks if the first and second elements in each tuple are different, resulting in the expected output [False, True, False].
The above is the detailed content of How Do Python's `any()` and `all()` Functions Work for Efficient Truthiness Evaluation of Iterables?. For more information, please follow other related articles on the PHP Chinese website!
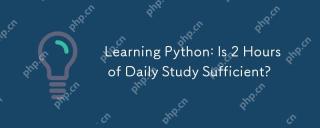
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
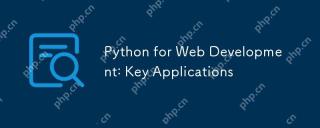
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
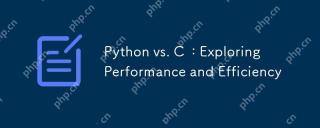
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
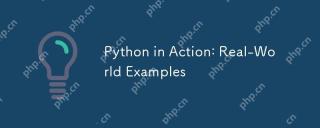
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
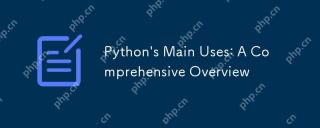
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
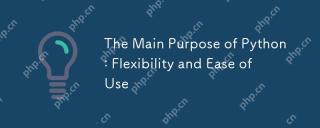
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
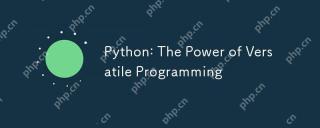
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
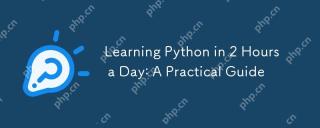
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
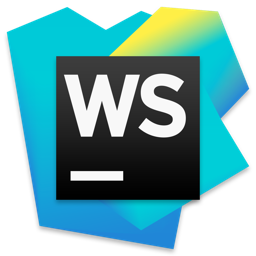
WebStorm Mac version
Useful JavaScript development tools
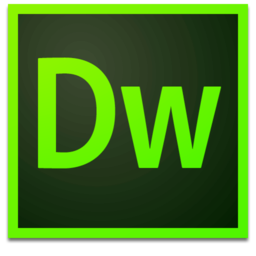
Dreamweaver Mac version
Visual web development tools
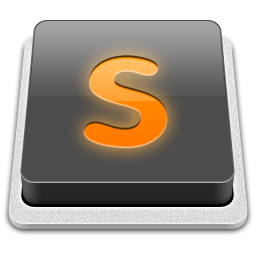
SublimeText3 Mac version
God-level code editing software (SublimeText3)