Custom Text Color in C# Console Application
In C#, it is possible to modify the text color in the console window. The default console colors are limited to the predefined ConsoleColor enumeration values, which do not include orange.
However, it is possible to set a custom text color by accessing the Windows API directly. To achieve this, you can use the SetConsoleScreenBufferInfoEx function to modify the color attributes of the console screen buffer.
Using the Native API
To set a specific console color to an RGB color, you can use the SetColor method in the SetScreenColorsApp class:
public static int SetColor(ConsoleColor color, uint r, uint g, uint b) { // Code to modify the console screen buffer info and set the specified color }
You can then use this method to set the text color to orange, for example:
SetColor(ConsoleColor.Gray, 255, 165, 0);
Using C# Extension Methods
Another approach is to use C# extension methods to simplify the process of setting custom text colors. Here's an example extension method that allows you to set the text color and background color using Color objects:
public static class ConsoleColorExtensions { public static void SetTextColor(this ConsoleColor[] color, Color foregroundColor) { // Code to set the foreground color } public static void SetBackgroundColor(this ConsoleColor[] color, Color backgroundColor) { // Code to set the background color } }
You can then use these extension methods to set the text color and background color as follows:
Console.SetTextColor(Color.Orange); Console.SetBackgroundColor(Color.Black);
Limitations
It's important to note that the ability to set custom text colors is limited to the Windows platform. If you are using C# on other platforms, you may need to explore platform-specific options to modify the text color.
The above is the detailed content of How to Set Custom Text Colors in a C# Console Application?. For more information, please follow other related articles on the PHP Chinese website!
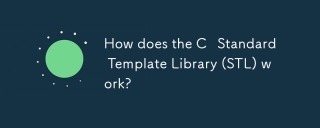
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
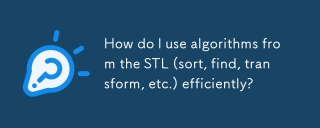
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
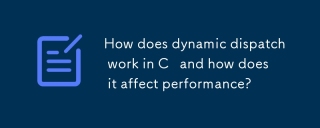
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
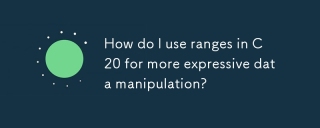
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
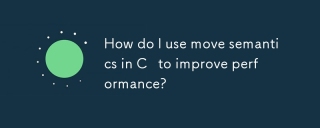
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
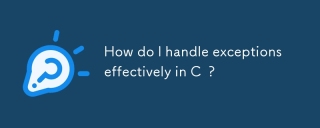
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
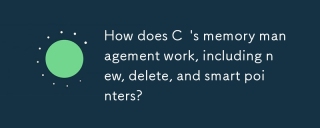
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
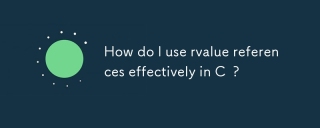
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
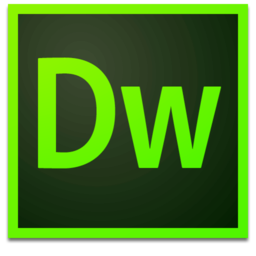
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
