Reading and Modifying NTFS Alternate Data Streams Using .NET
NTFS Alternate Data Streams (ADS) are hidden data streams associated with regular files in the New Technology File System (NTFS). These streams can be used to store additional information, such as user comments, version history, or multimedia content, without affecting the primary file data.
Reading ADS
To read an ADS, you can use the CreateFileW function with the dwDesiredAccess parameter set to GENERIC_WRITE. This will open the stream for both reading and writing. You can then use the ReadFile function to read the stream's contents.
Modifying ADS
To modify an ADS, you can use the CreateFileW function with the dwDesiredAccess parameter set to GENERIC_WRITE. This will open the stream for both reading and writing. You can then use the WriteFile function to write new contents to the stream.
Here is a C# example of how to read and modify an ADS:
using System.Runtime.InteropServices; class Program { static void Main(string[] args) { // Open the main file stream var mainStream = NativeMethods.CreateFileW( "testfile", NativeConstants.GENERIC_WRITE, NativeConstants.FILE_SHARE_WRITE, IntPtr.Zero, NativeConstants.OPEN_ALWAYS, 0, IntPtr.Zero); // Open the ADS stream var stream = NativeMethods.CreateFileW( "testfile:stream", NativeConstants.GENERIC_WRITE, NativeConstants.FILE_SHARE_WRITE, IntPtr.Zero, NativeConstants.OPEN_ALWAYS, 0, IntPtr.Zero); // Write data to the ADS stream var data = "Hello world!"; NativeMethods.WriteFile(stream, data, data.Length, out var bytesWritten, IntPtr.Zero); // Close the ADS stream NativeMethods.CloseHandle(stream); // Close the main file stream NativeMethods.CloseHandle(mainStream); } } public partial class NativeMethods { /// Return Type: HANDLE->void* ///lpFileName: LPCWSTR->WCHAR* ///dwDesiredAccess: DWORD->unsigned int ///dwShareMode: DWORD->unsigned int ///lpSecurityAttributes: LPSECURITY_ATTRIBUTES->_SECURITY_ATTRIBUTES* ///dwCreationDisposition: DWORD->unsigned int ///dwFlagsAndAttributes: DWORD->unsigned int ///hTemplateFile: HANDLE->void* [DllImportAttribute("kernel32.dll", EntryPoint = "CreateFileW")] public static extern System.IntPtr CreateFileW( [InAttribute()] [MarshalAsAttribute(UnmanagedType.LPWStr)] string lpFileName, uint dwDesiredAccess, uint dwShareMode, [InAttribute()] System.IntPtr lpSecurityAttributes, uint dwCreationDisposition, uint dwFlagsAndAttributes, [InAttribute()] System.IntPtr hTemplateFile ); /// Return Type: BOOL->int ///hFile: HANDLE->void* ///lpBuffer: LPVOID->void* ///nNumberOfBytesToWrite: DWORD->unsigned int ///lpNumberOfBytesWritten: LPDWORD->DWORD* ///lpOverlapped: LPOVERLAPPED->_OVERLAPPED* [DllImportAttribute("kernel32.dll", EntryPoint = "WriteFile")] public static extern int WriteFile( System.IntPtr hFile, [InAttribute()] System.IntPtr lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [InAttribute()] System.IntPtr lpOverlapped ); /// Return Type: BOOL->int ///hObject: HANDLE->void* [DllImportAttribute("kernel32.dll", EntryPoint = "CloseHandle")] public static extern int CloseHandle( [InAttribute()] System.IntPtr hObject ); } public partial class NativeConstants { /// GENERIC_WRITE -> (0x40000000L) public const int GENERIC_WRITE = 1073741824; /// FILE_SHARE_DELETE -> 0x00000004 public const int FILE_SHARE_DELETE = 4; /// FILE_SHARE_WRITE -> 0x00000002 public const int FILE_SHARE_WRITE = 2; /// FILE_SHARE_READ -> 0x00000001 public const int FILE_SHARE_READ = 1; /// OPEN_ALWAYS -> 4 public const int OPEN_ALWAYS = 4; }
The above is the detailed content of How Can I Read and Modify NTFS Alternate Data Streams Using .NET?. For more information, please follow other related articles on the PHP Chinese website!
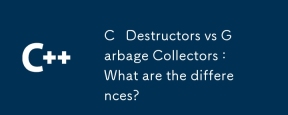
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
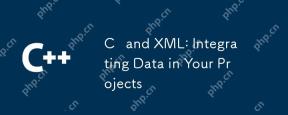
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
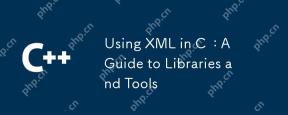
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
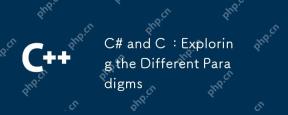
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
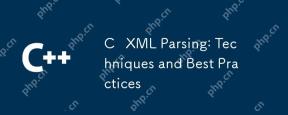
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
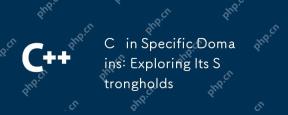
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
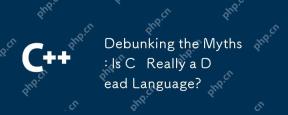
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
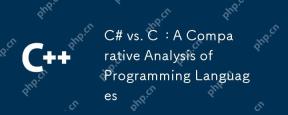
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
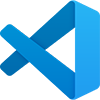
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
