Bulk Inserting Parameterized Variables into a Database in C
In C#, inserting multiple rows into a database can be a time-consuming process if each row requires a separate INSERT statement. To optimize performance, it is desirable to insert multiple rows in a single query while also utilizing parameterized queries to prevent SQL injection attacks.
To achieve this, you can leverage the power of table-valued parameters, which allow you to pass a structured data type (such as a DataTable) to a stored procedure. This enables you to insert multiple rows with a single command.
To implement this approach, follow these steps:
1. Create a User-Defined Table Type (UDT)
Create a UDT to represent the rows you want to insert. For example, if you have columns "Col1" and "Col2", you can create a UDT as follows:
CREATE TYPE MyTableType AS TABLE ( Col1 int, Col2 varchar(20) ) GO
2. Create a Stored Procedure
Create a stored procedure to accept the UDT as a parameter:
CREATE PROCEDURE MyProcedure ( @MyTable dbo.MyTableType READONLY -- Table valued parameters must be ReadOnly! ) AS INSERT INTO MyTable (Col1, Col2) SELECT Col1, Col2 FROM @MyTable GO
3. Execute the Stored Procedure from C
Populate a DataTable with your data:
DataTable dt = new DataTable(); dt.Columns.Add("Col1", typeof(int)); dt.Columns.Add("Col2", typeof(string)); // Populate data
Open a connection and execute the stored procedure using a SqlCommand:
using (var con = new SqlConnection("ConnectionString")) { using(var cmd = new SqlCommand("MyProcedure", con)) { cmd.CommandType = CommandType.StoredProcedure; cmd.Parameters.Add("@MyTable", SqlDbType.Structured).Value = dt; con.Open(); cmd.ExecuteNonQuery(); } }
By utilizing table-valued parameters and stored procedures, you can significantly improve the performance of bulk inserts, while also maintaining the security and efficiency of parameterized queries.
The above is the detailed content of How Can I Efficiently Bulk Insert Data with Parameterized Queries in C#?. For more information, please follow other related articles on the PHP Chinese website!
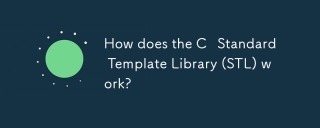
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
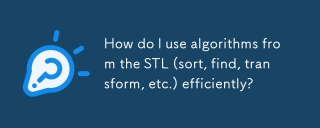
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
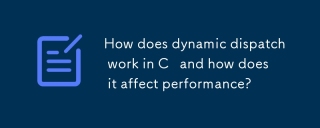
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
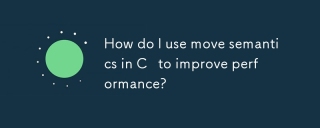
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
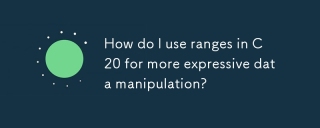
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
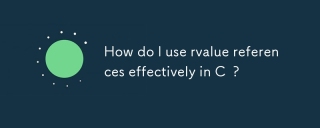
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
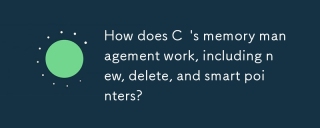
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
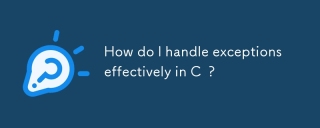
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
