Adding Attributes to Properties Dynamically
This code sample demonstrates how to add validation attributes to properties at runtime. It leverages reflection to access the property's FillAttributes method and inject the desired attribute.
Code Analysis
// Get PropertyDescriptor object for the given property name var propDesc = TypeDescriptor.GetProperties(typeof(T))[propName]; // Get FillAttributes methodinfo delegate var methodInfo = propDesc.GetType().GetMethods(BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic) .FirstOrDefault(m => m.IsFamily || m.IsPublic && m.Name == "FillAttributes"); // Create Validation attribute var attribute = new RequiredAttribute(); var attributes = new ValidationAttribute[] { attribute }; // Invoke FillAttribute method methodInfo.Invoke(propDesc, new object[] { attributes });
Exception Analysis
However, upon execution, you may encounter the "Collection was of a fixed size" exception. This occurs because the property's validation attributes collection is defined as a fixed-size array. To address this, we need to replace the existing array with a new, larger one.
Solution
To resolve this issue, you can implement a custom attribute caching system to store and apply attributes dynamically. An example implementation could be:
// Initialize cache dictionary and synchronization mechanism private static readonly Dictionary<type attribute> AttributeCache = new Dictionary<type attribute>(); private static readonly object SyncObject = new object(); protected override Attribute[] GetCustomAttributes(Type attributeType, bool inherit) { if (AttributeCache.TryGetValue(this.GetType(), out Attribute[] cachedAttrs)) { return cachedAttrs; } else { lock (SyncObject) { if (!AttributeCache.TryGetValue(this.GetType(), out cachedAttrs)) { cachedAttrs = base.GetCustomAttributes(attributeType, inherit); AttributeCache.Add(this.GetType(), cachedAttrs); } } return cachedAttrs; } }</type></type>
With this custom caching mechanism, the code will successfully add validation attributes to properties at runtime without causing the fixed-size collection exception.
The above is the detailed content of How Can I Dynamically Add Validation Attributes to Properties in C#?. For more information, please follow other related articles on the PHP Chinese website!
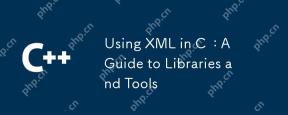
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
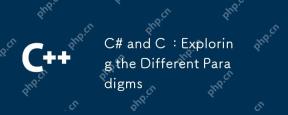
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
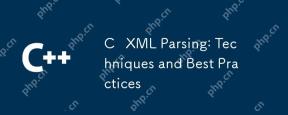
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
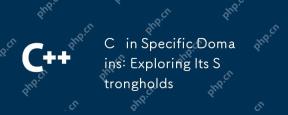
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
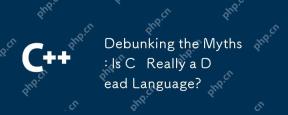
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
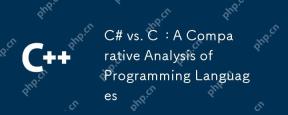
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
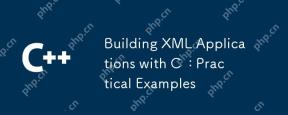
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
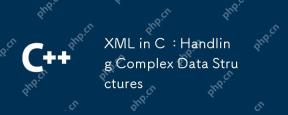
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
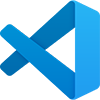
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
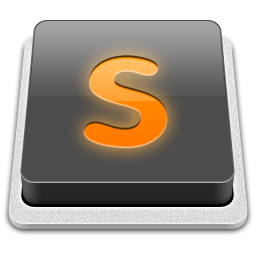
SublimeText3 Mac version
God-level code editing software (SublimeText3)
