Function Pointer Dereferencing: Unraveling the Mystery
In C programming, a function pointer is a variable that stores the address of a function. However, unlike regular variables, dereferencing a function pointer behaves uniquely.
Why does dereferencing a function pointer not produce the expected result? The key lies in understanding how function values operate in rvalue contexts (where they are used as values rather than as locations). In C, function values in rvalue contexts are automatically converted to pointers to their original function value.
Upon dereferencing this pointer with *, the original function value is retrieved. However, this value is immediately reconverted to a pointer, creating an infinite loop of pointer conversions. The code provided in the question illustrates this behavior:
#include <stdio.h> void hello() { printf("hello"); } int main(void) { (*****hello)(); }</stdio.h>
This code essentially calls the hello function five times through a chain of function pointer dereferences. However, these dereferences do not actually execute the function; they merely retrieve the function pointer, which is then converted back to a pointer. The end result is a series of pointer manipulations, but no actual function call.
To understand why this happens, consider a similar experiment:
int x; // Regular variable int *px = &x; // Pointer to the variable *px = 5; // Modifying the variable through the pointer
In this code, dereferencing the pointer *px allows us to modify the value of the variable x. However, in the case of a function pointer, dereferencing does not modify the function itself, but rather retrieves its address.
This distinction exists because functions are immutable in C, meaning they cannot be modified. They can only be called or passed around as pointers. Therefore, there is no need for dereferencing a function pointer to modify its behavior.
In summary, dereferencing a function pointer does not execute the function, but rather retrieves its address. This address is then immediately reconverted to a pointer, resulting in an infinite loop of pointer conversions. This behavior is a unique feature of function values in rvalue contexts in C and serves as a convenience for working with function pointers without having to explicitly use ampersand (&) for address referencing.
The above is the detailed content of Why Doesn't Dereferencing a Function Pointer in C Execute the Function?. For more information, please follow other related articles on the PHP Chinese website!
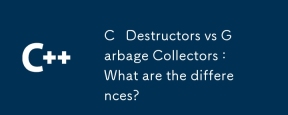
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
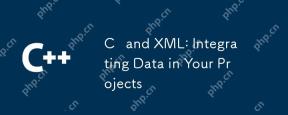
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
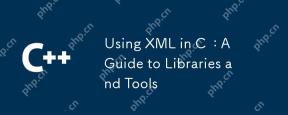
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
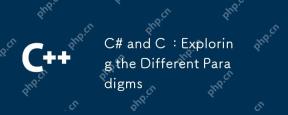
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
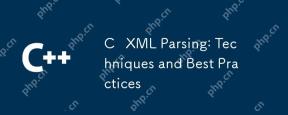
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
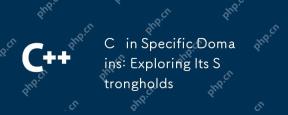
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
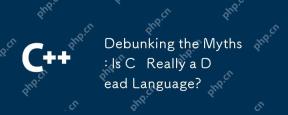
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
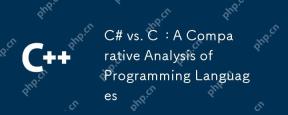
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
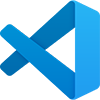
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
