


FastAPI: Understanding and Resolving Error 422 when Sending JSON Data via POST Request
Problem Description
Building an API with FastAPI, the application exhibits error code 422 (Unprocessable Entity) while sending JSON data through a POST request. GET requests, on the other hand, work smoothly. This issue persists despite attempts to parse JSON, use UTF-8 encoding, or modify HTTP headers.
Detailed Explanation
A response with the 422 status code indicates an error with the submitted data, often due to missing or incorrectly formatted elements. In this case, the problem stems from the expected format of the POST request. By default, FastAPI anticipates user input as a query parameter, not as JSON payload. Consequently, the client's attempt to transmit JSON data results in the 422 error.
Solution Options
The following four options offer distinct approaches to defining an endpoint that correctly processes JSON data from a POST request:
1. Using Pydantic Models
Employing Pydantic models enables you to specify an expected data structure for your endpoint.
from pydantic import BaseModel class User(BaseModel): user: str @app.post('/') def main(user: User): return user
2. Using the Body Parameter Embed
This method utilizes the special "embed" parameter to treat the body as a single parameter.
from fastapi import Body @app.post('/') def main(user: str = Body(..., embed=True)): return {'user': user}
3. Using a Dict Type (Less Recommended)
While less recommended, this approach defines a key-value pair as a Dict type.
from typing import Dict, Any @app.post('/') def main(payload: Dict[Any, Any]): return payload
4. Directly Using the Request Object
This option involves using the Request object to parse the received JSON data.
from fastapi import Request @app.post('/') async def main(request: Request): return await request.json()
Testing the Solutions
To test the provided solutions, follow the given steps:
Using Python Requests Library
import requests url = 'http://127.0.0.1:8000/' payload ={'user': 'foo'} resp = requests.post(url=url, json=payload) print(resp.json())
Using JavaScript Fetch API
fetch('/', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({'user': 'foo'}) }) .then(resp => resp.json()) .then(data => { console.log(data); }) .catch(error => { console.error(error); });
The above is the detailed content of FastAPI POST Request Error 422: How to Properly Handle JSON Data?. For more information, please follow other related articles on the PHP Chinese website!
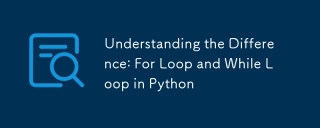
ThedifferencebetweenaforloopandawhileloopinPythonisthataforloopisusedwhenthenumberofiterationsisknowninadvance,whileawhileloopisusedwhenaconditionneedstobecheckedrepeatedlywithoutknowingthenumberofiterations.1)Forloopsareidealforiteratingoversequence
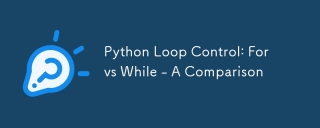
In Python, for loops are suitable for cases where the number of iterations is known, while loops are suitable for cases where the number of iterations is unknown and more control is required. 1) For loops are suitable for traversing sequences, such as lists, strings, etc., with concise and Pythonic code. 2) While loops are more appropriate when you need to control the loop according to conditions or wait for user input, but you need to pay attention to avoid infinite loops. 3) In terms of performance, the for loop is slightly faster, but the difference is usually not large. Choosing the right loop type can improve the efficiency and readability of your code.
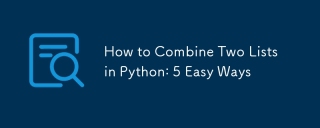
In Python, lists can be merged through five methods: 1) Use operators, which are simple and intuitive, suitable for small lists; 2) Use extend() method to directly modify the original list, suitable for lists that need to be updated frequently; 3) Use list analytical formulas, concise and operational on elements; 4) Use itertools.chain() function to efficient memory and suitable for large data sets; 5) Use * operators and zip() function to be suitable for scenes where elements need to be paired. Each method has its specific uses and advantages and disadvantages, and the project requirements and performance should be taken into account when choosing.
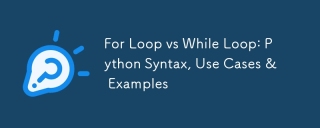
Forloopsareusedwhenthenumberofiterationsisknown,whilewhileloopsareuseduntilaconditionismet.1)Forloopsareidealforsequenceslikelists,usingsyntaxlike'forfruitinfruits:print(fruit)'.2)Whileloopsaresuitableforunknowniterationcounts,e.g.,'whilecountdown>
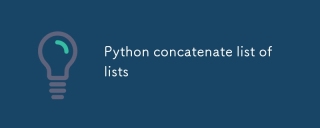
ToconcatenatealistoflistsinPython,useextend,listcomprehensions,itertools.chain,orrecursivefunctions.1)Extendmethodisstraightforwardbutverbose.2)Listcomprehensionsareconciseandefficientforlargerdatasets.3)Itertools.chainismemory-efficientforlargedatas
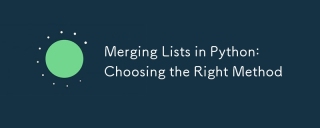
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
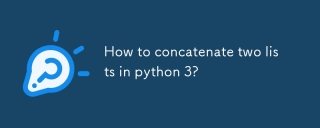
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
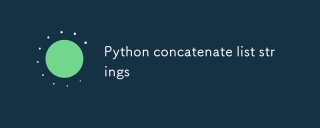
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
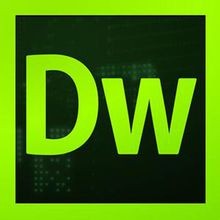
Dreamweaver CS6
Visual web development tools
