How to Decode Streaming JSON in Go
When working with large JSON responses, it's not ideal to load the entire response into memory before decoding it. Using the ioutil.ReadAll function can lead to memory issues when dealing with large JSON payloads. This article will explore how to decode JSON data on the fly as it streams in, avoiding memory consumption problems.
JSON Streaming with json.Decoder
The json.Decoder in the Go standard library provides the ability to parse JSON streams incrementally. This is achieved through the Decoder.Token() method.
The Decoder.Token() method returns the next token in the JSON stream without consuming it. This allows for selective parsing of JSON data and event-driven processing.
Handling JSON Structures
Event-driven parsing requires a state machine to track the current position within the JSON structure. We can use this state machine to process different parts of the JSON data as they appear in the stream.
For example, let's say we receive a JSON response with the following format:
{ "property1": "value1", "property2": "value2", "array": [ { "item1": "value3" }, { "item2": "value4" } ] }
We can write a function that incrementally parses this JSON stream and processes the array element separately:
func processJSONStream(stream io.Reader) { decoder := json.NewDecoder(stream) state := "start" for decoder.More() { token, err := decoder.Token() if err != nil { log.Fatal(err) } switch state { case "start": if delim, ok := token.(json.Delim); ok && delim == '{' { state = "object" } else { log.Fatal("Expected object") } case "object": switch t := token.(type) { case json.Delim: if t == '}' { // End of object state = "end" } else if t == ',' { // Next property continue } else if t == '[' { // Array found state = "array" } if t == ':' { // Property value expected state = "prop_value" } case string: // Property name fmt.Printf("Property '%s'\n", t) default: // Property value fmt.Printf("Value: %v\n", t) } case "array": if delim, ok := token.(json.Delim); ok && delim == ']' { // End of array state = "object" } else if token == json.Delim('{') { // Array item object fmt.Printf("Item:\n") state = "item" } case "item": switch t := token.(type) { case json.Delim: if t == '}' { // End of item object fmt.Printf("\n") state = "array" } else if t == ',' { // Next item property fmt.Printf(",\n") continue } case string: // Item property name fmt.Printf("\t'%s'", t) default: // Item property value fmt.Printf(": %v", t) } case "prop_value": // Decode the property value var value interface{} if err := decoder.Decode(&value); err != nil { log.Fatal(err) } fmt.Printf("Value: %v\n", value) state = "object" } } }
When called with the JSON response, this function will print the property names and values, as well as the individual items within the array.
Conclusion
Using the json.Decoder and Decoder.Token() in event-driven processing allows us to parse large JSON responses incrementally, avoiding memory consumption issues and enabling efficient processing of data as it streams in.
The above is the detailed content of How to Efficiently Decode Large Streaming JSON in Go?. For more information, please follow other related articles on the PHP Chinese website!
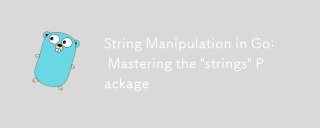
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
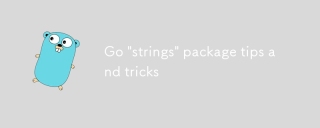
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
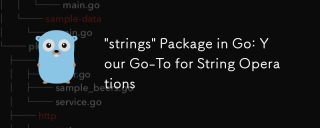
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
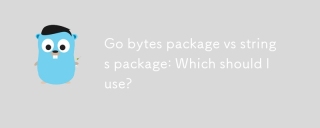
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
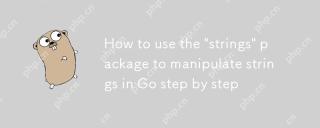
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
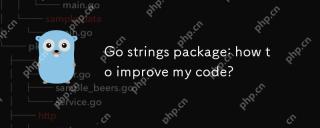
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
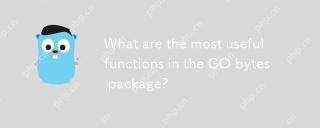
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
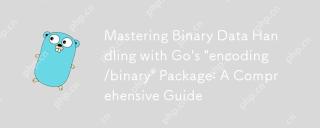
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
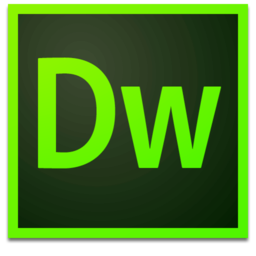
Dreamweaver Mac version
Visual web development tools
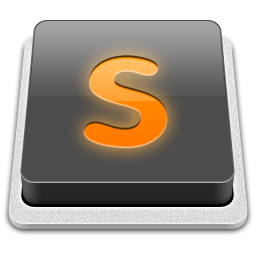
SublimeText3 Mac version
God-level code editing software (SublimeText3)
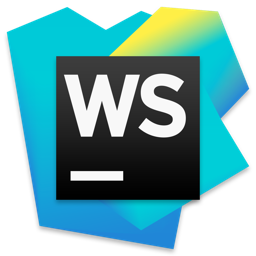
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
