


How Can I Resolve Function Hiding Issues When Overloading Functions in C Inheritance?
Function Overloading in Inheritance: Understanding Hidden Functions
In object-oriented programming, inheritance allows classes to inherit properties and methods from parent classes. However, when defining functions with the same name but different signatures in base and derived classes, name lookup can become ambiguous, leading to errors.
Problem:
Consider the following code snippet:
class A { public: void foo(string s){}; }; class B : public A { public: int foo(int i){}; }; class C : public B { public: void bar() { string s; foo(s); // Error: member function not found } };
When attempting to call the overridden foo function within the derived class C, the compiler encounters an error because name lookup stops at the derived class B and cannot find the inherited foo from the base class A.
Solution:
To resolve this issue, the function from the base class must be explicitly declared in the derived class's scope using the using keyword. This makes both functions visible from within the derived class.
class A { public: void foo(string s){}; }; class B : public A { public: int foo(int i){}; using A::foo; // Re-declare function from base class }; class C : public B { public: void bar() { string s; foo(s); // Now calls the inherited 'foo' function } };
Explanation:
The name lookup in inheritance is hierarchical. By default, name lookup prioritizes functions defined in the derived class. In the above example, when foo(s) is called in C, the compiler first looks for a matching function in C, then in B, and finally in A. However, since B has its own foo function, the lookup stops there, resulting in the error.
By explicitly declaring the base class's foo function in B using using A::foo, the compiler is instructed to consider both functions during name lookup. This allows the inherited foo function to be found and invoked from C.
In essence, this technique solves the problem of function hiding in derived classes and ensures correct name lookup when working with overloaded functions in inheritance hierarchies.
The above is the detailed content of How Can I Resolve Function Hiding Issues When Overloading Functions in C Inheritance?. For more information, please follow other related articles on the PHP Chinese website!
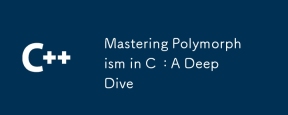
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
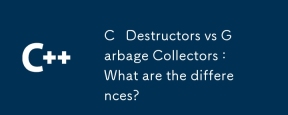
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
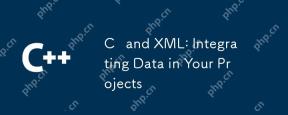
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
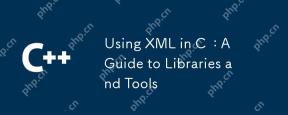
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
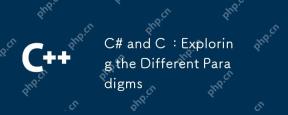
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
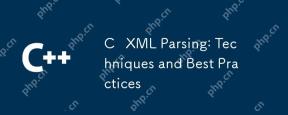
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
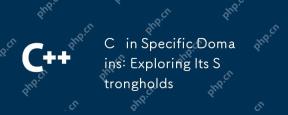
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
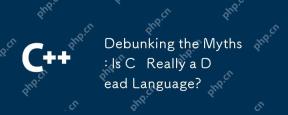
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
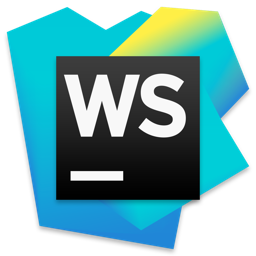
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
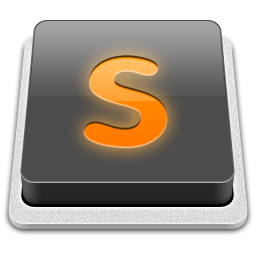
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
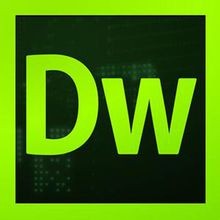
Dreamweaver CS6
Visual web development tools
