


Separating Class and Member Functions into Header and Source Files
In C , classes can be declared and implemented in separate header and source files. This allows for easier management and organization of code.
Class Declaration in Header File:
The header file (.h) contains the class declaration, which includes the name, data members, and member function prototypes. Include guards are used to prevent multiple inclusions.
// A2DD.h #ifndef A2DD_H #define A2DD_H class A2DD { public: A2DD(int x, int y); int getSum(); }; #endif
Class Implementation in Source File:
The source file (.cpp) contains the implementation of the class's member functions. The functions are defined using the class's scope operator (::).
// A2DD.cpp #include "A2DD.h" A2DD::A2DD(int x, int y) { gx = x; gy = y; } int A2DD::getSum() { return gx + gy; }
Syntax for Using the Class:
To use the class, include the header file in the main file. Instantiation of the class and access to its member functions is done as follows:
// main.cpp #include "A2DD.h" int main() { A2DD a(1, 2); int sum = a.getSum(); return 0; }
The above is the detailed content of How to Separate C Class Declarations and Member Function Implementations into Header and Source Files?. For more information, please follow other related articles on the PHP Chinese website!
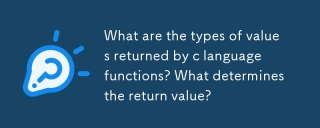
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
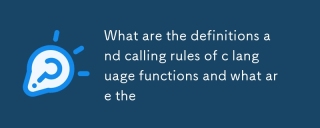
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi
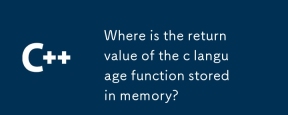
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
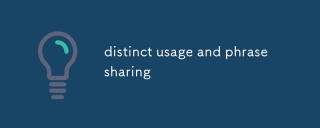
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
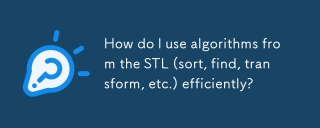
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
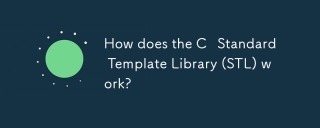
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
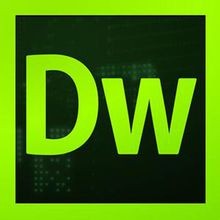
Dreamweaver CS6
Visual web development tools
