Method Overloading with Null Argument: Resolving Ambiguity
In Java, method overloading allows multiple methods with the same name but different parameter types. However, passing a null argument to overloaded methods can lead to compiler errors. Let's explore the issue and its solution.
Consider the following code snippet:
public static void doSomething(Object obj) { System.out.println("Object called"); } public static void doSomething(char[] obj) { System.out.println("Array called"); } public static void doSomething(Integer obj) { System.out.println("Integer called"); }
Invoking doSomething(null) results in a compilation error due to method ambiguity. Java cannot determine which version of doSomething to call since Integer, char[], and Object can all accept null values.
The issue lies with the equally specific nature of the Integer and char[] methods. Both are more specific than the Object method because they define a narrower parameter type. Therefore, Java cannot prioritize one method over the other.
To resolve this ambiguity, you must explicitly specify the intended method by casting the argument to the desired type. For instance:
doSomething((Object) null); // Calls doSomething(Object obj) doSomething((char[]) null); // Calls doSomething(char[] obj)
Alternatively, you can make the parameter type non-nullable in one of the overloaded methods. This would ensure that null cannot be passed as an argument to that version. For example:
public static void doSomething(Object obj) { System.out.println("Object called"); } public static void doSomething(char[] obj) { // Parameter type not nullable System.out.println("Array called"); }
By casting the argument or making the parameter type non-nullable, you provide Java with additional information to disambiguate the method call.
The above is the detailed content of How to Resolve Method Overloading Ambiguity with Null Arguments in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
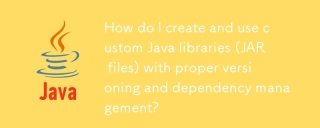
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
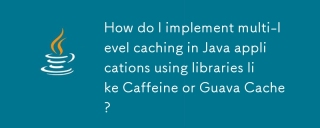
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
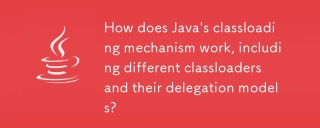
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
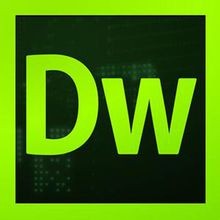
Dreamweaver CS6
Visual web development tools
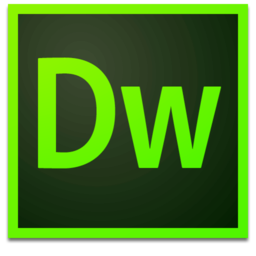
Dreamweaver Mac version
Visual web development tools