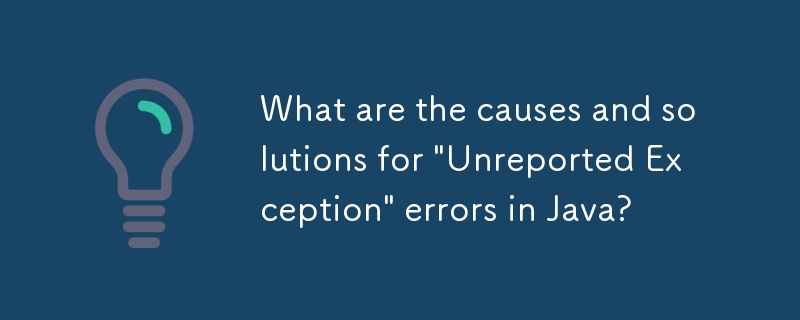
Unreported Exception: Meaning and Resolution
Java programmers often encounter errors framed as follows:
"error: unreported exception where XXX is the name of some exception class."
Meaning and Java Concepts
This error signifies that:
- The code is throwing or propagating a checked exception (XXX).
- The exception is not being properly handled by catching it or declaring it as required by Java.
Resolution
To fix the issue, you have two options:
-
Catch the Exception: Use a try ... catch block to handle the exception within the current method.
-
Declare that the Method Throws the Exception: Add the throws keyword followed by the name of the exception in the method or constructor signature to pass the exception handling responsibility to the caller.
Checked vs Unchecked Exceptions
Java classifies exceptions into two types:
-
Checked Exceptions: Throwable, Exception (and subclasses), excluding RuntimeException and its subclasses.
-
Unchecked Exceptions: Error (and subclasses), RuntimeException (and subclasses).
Checked exceptions require explicit handling, while unchecked exceptions do not.
Example: Throwing and Catching in the Same Method
static void t() throws IllegalAccessException {
try {
throw new IllegalAccessException("demo");
} catch (IllegalAccessException e) {
System.out.println(e);
}
}
In this example, the t method throws an exception, but it is also caught and handled within the same method. Hence, the throws declaration in the signature should be removed.
Bad Practices with Exceptions
Avoid the following bad practices:
-
Catching Exception (or Throwable): Catch specific exceptions instead to prevent unintended handling.
-
Declaring Methods as Throwing Exception: Avoid forcing callers to handle potentially any checked exception.
-
Squashing Exceptions: Don't ignore exceptions because they can make runtime errors harder to diagnose.
Special Cases
-
Static Initializers: Declare static variables throwing checked exceptions in static blocks instead of field declarations.
-
Static Blocks: Caught exceptions within static blocks must be handled inside the block because there is no enclosing context.
-
Lambdas: Lambdas cannot throw unchecked exceptions due to the function interface requirements. Handle exceptions within the lambda itself.
-
Two Exceptions with Same Name: If the compiler reports an "unreported exception" error despite the presence of the throws keyword, check if the exception names have different fully qualified names, indicating different exceptions.
Further Information
Refer to the following resources for detailed information on Java exceptions:
- [Oracle Java Tutorial: The catch or specify requirement](https://docs.oracle.com/javase/tutorial/essential/exceptions/catchOrDeclare.html)
- [Catching and handling exceptions](https://docs.oracle.com/javase/tutorial/essential/exceptions/handling.html)
- [Specifying the exceptions thrown by a method](https://docs.oracle.com/javase/tutorial/essential/exceptions/throwing.html)
The above is the detailed content of What are the causes and solutions for 'Unreported Exception' errors in Java?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn