


How to Find the Closest Color in a Color Array Using Different Distance Metrics?
How to Determine the Closest Color in an Array of Colors
Introduction
Finding the most similar color within an array can be a useful technique in various applications. This question explores three methods to measure color similarity and retrieve the index of the closest match.
Methods
Method 1: Hue Comparison
When considering only hue (the color shade), this method finds the closest hue to the target color. It ignores saturation and brightness.
int closestColor1(List<color> colors, Color target) { var hue1 = target.GetHue(); var diffs = colors.Select(n => getHueDistance(n.GetHue(), hue1)); var diffMin = diffs.Min(n => n); return diffs.ToList().FindIndex(n => n == diffMin); }</color>
Method 2: RGB Space Distance
This method directly measures the difference in RGB values between the target color and each color in the array.
int closestColor2(List<color> colors, Color target) { var colorDiffs = colors.Select(n => ColorDiff(n, target)).Min(n => n); return colors.FindIndex(n => ColorDiff(n, target) == colorDiffs); }</color>
Method 3: Weighted Distance
This method incorporates hue, saturation, and brightness to gauge the similarity. The hue component has a higher weight by default.
int closestColor3(List<color> colors, Color target) { float hue1 = target.GetHue(); var num1 = ColorNum(target); var diffs = colors.Select(n => Math.Abs(ColorNum(n) - num1) + getHueDistance(n.GetHue(), hue1)); var diffMin = diffs.Min(x => x); return diffs.ToList().FindIndex(n => n == diffMin); }</color>
Helper Functions
- getBrightness: Calculates the perceived color brightness.
- getHueDistance: Determines the hue difference between two colors.
- ColorNum: Weighs the saturation and brightness components.
- ColorDiff: Computes the RGB color space distance.
Usage
int indexInArray = closestColor1(clist.ToList(), someColor);
Conclusion
The choice of method depends on the specific requirements of the application. For hue-based comparisons, Method 1 is sufficient. Method 2 measures the RGB distance, while Method 3 provides a weighted distance calculation considering all color properties.
The above is the detailed content of How to Find the Closest Color in a Color Array Using Different Distance Metrics?. For more information, please follow other related articles on the PHP Chinese website!
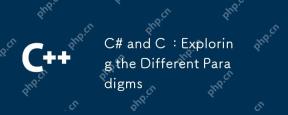
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
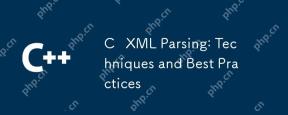
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
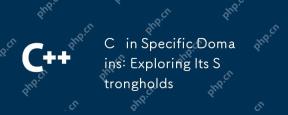
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
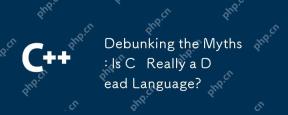
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
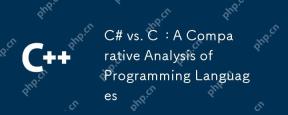
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
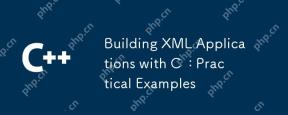
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
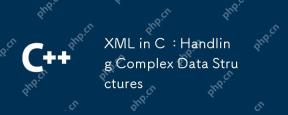
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
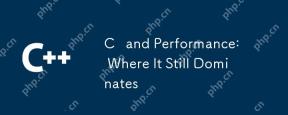
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
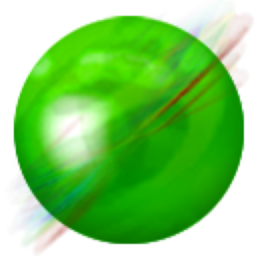
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
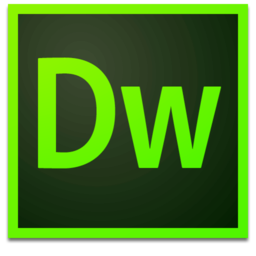
Dreamweaver Mac version
Visual web development tools
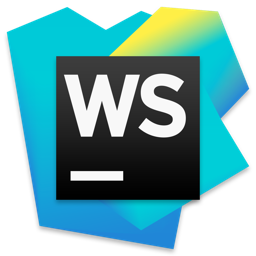
WebStorm Mac version
Useful JavaScript development tools
