Introduction
In this post, I'll walk you through why Playwright and Cucumber are exceptional tools for end-to-end (E2E) testing. We'll then dive into the steps for integrating Playwright for seamless usage in a JavaScript frontend application. Finally, I'll share some pro tips to maximize your efficiency with Playwright.
Why Playwright and Cucumber?
While there are numerous capable tools for building E2E test suites, none match the synergy of Playwright and Cucumber.
Here’s why Playwright stands out in the market to write E2E tests, to name a few:
- 1. Any browser, Any platform, One API
- a. Cross-browser. Playwright supports all modern rendering engines including Chromium, WebKit, and Firefox.
- b. Cross-platform. Test on Windows, Linux, and macOS, locally or on CI, headless or headed.
- c. Cross-language. Use the Playwright API in TypeScript, JavaScript, Python, .NET, and Java.
- 2. Resilient, No flaky tests
- a. Auto-wait. The playwright waits for elements to be actionable before performing actions.
- b. Web-first assertions. Playwright assertions are created specifically for the dynamic web.
- 3. Powerful Tooling
- a. Codegen. Generate tests by recording your actions. Save them in any language.
- b. Playwright inspector. Inspect the page, generate selectors, step through the test execution, see click points, and explore execution logs.
However, without Cucumber managing test code can become a maintenance nightmare. Cucumber facilitates writing tests in plain, human-readable language, making them accessible even to non-technical stakeholders. As a cornerstone of Behavior Driven Development (BDD), Cucumber acts as technical documentation and accelerates onboarding for new engineers.
Integration of Playwright and Cucumber
Step 1: Install Dependencies
Install the necessary packages using npm or yarn.
npm install @playwright/test playwright-core @cucumber/cucumber cucumber-html-reporter concurrently
Step 2: Setup Project Structure
Organize your project like this:
cucumber.js cucumber.report.js /e2e /features example.feature /steps example.steps.js
Step 3: Configure Cucumber
Create a Cucumber Configuration File - cucumber.js file in the root of your project with the following content:
// filepath: /cucumber.js module.exports = { default: { require: ["./steps/**/*.js"], format: ["pretty"], paths: ["./features/**/*.feature"], }, };
Step 4: Configure Test Reporting
Create cucumber.report.js - A file to configure the test report. Configuration options can be found here
// filepath: /cucumber.report.js import reporter from 'cucumber-html-reporter const options = { theme: 'bootstrap', output: report/report.html', jsonFile: 'report/report.json', brandTitle: 'E2E Test Report' };
Step 5: Write a Feature File
Create a feature file in the features directory, e.g., example.feature:
npm install @playwright/test playwright-core @cucumber/cucumber cucumber-html-reporter concurrently
Step 6: Write Step Definitions
Create a step definition file in the steps directory, e.g., example.steps.js:
cucumber.js cucumber.report.js /e2e /features example.feature /steps example.steps.js
Step 7: Add a NPM Test Script
Add a script to your package.json to run the tests:
// filepath: /cucumber.js module.exports = { default: { require: ["./steps/**/*.js"], format: ["pretty"], paths: ["./features/**/*.feature"], }, };
concurrently npm package is a handy tool that lets you execute two processes at the same test, as to execute the E2E test you'll need to run the application as well, sometimes locally and sometimes remotely.
Step 8: Run Tests
Execute tests with:
// filepath: /cucumber.report.js import reporter from 'cucumber-html-reporter const options = { theme: 'bootstrap', output: report/report.html', jsonFile: 'report/report.json', brandTitle: 'E2E Test Report' };
Playwright Pro Tips
- 1. Error Messages - Read them carefully—they often pinpoint the problem.
- 2. Iterative Development - Test small chunks of code to isolate and resolve issues.
- 3. Playwright Inspector - It can help to record actions and generate test scripts automatically, so would have to write just a piece of code. You can launch Playwright Inspector as below
// filepath: /e2e/features/example.feature Feature: Example feature Scenario: Open a webpage Given I open the "https://example.com" page Then the title should be "Example.com"
- 4. Log Console Messages - Print browser console message in headless mode as below
// filepath: /e2e/steps/example.steps.js import { Before, Given, Then, setDefaultTimeout, After } from '@cucumber/cucumber'; import { chromium, expect } from 'playwright'; setDefaultTimeout(30 * 1000); //milliseconds let browser, page, context; Before(async function(){ browser = await chromium.launch({headless: true}); context = await brwoser.newContext(); page = await content.newPage(); }); Given('I open the {string} page', async function (url) { await page.goto(url); }); Then('the title should be {string}', async function (title) { const pageTitle = await page.title(); expect(pageTitle).toHaveText(title); }); After(async function(){ await context.close(); await browser.close(); });
- 5. Handle Lazy Loading - For lazy loading elements, use the following functions
- a. waitForSelector or waitFor
- b. waitForTimeout
- c. scrollIntoViewIfNeeded
- 6. Debug DOM - Use document.querySelector or other query selector in the browser console to locate the elements
- 7. Capture a screenshot of the failure
If you have reached here, then I made a satisfactory effort to keep you reading. Please be kind enough to leave any comments or share corrections.
My Other Blogs:
- Integrate Web Component/MFE with plain static HTML
- Cracking Software Engineering Interviews
- My firsthand experience with web component - learnings and limitations
- Micro-Frontend Decision Framework
- Test SOAP Web Service using Postman Tool
The above is the detailed content of Supercharge Your ETests with Playwright and Cucumber Integration. For more information, please follow other related articles on the PHP Chinese website!
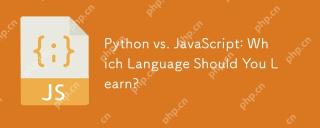
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
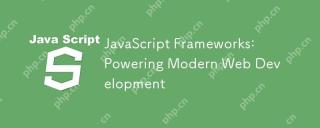
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
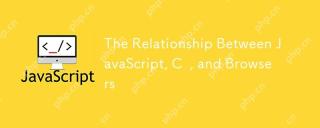
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
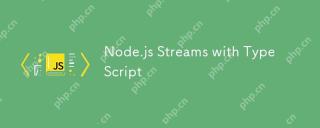
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
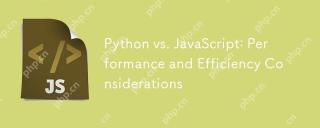
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
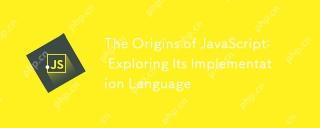
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
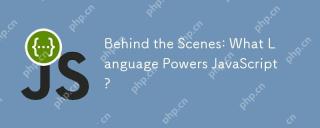
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
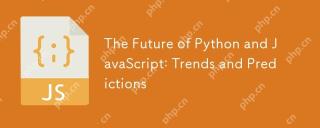
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
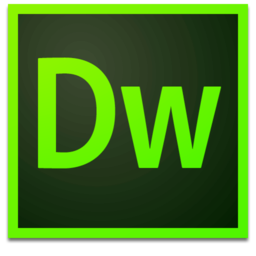
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
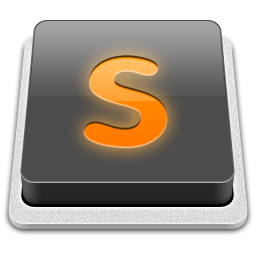
SublimeText3 Mac version
God-level code editing software (SublimeText3)
