Creating a Telegram bot that integrates with an AI assistant is an exciting project that combines real-time messaging, AI processing, and Golang's powerful concurrency model. In this blog, I'll guide you through the architecture, features, and implementation of a Telegram bot using Golang, with a complete system design to boot!
Overview
This project builds a personal AI assistant accessible via two interfaces:
Telegram Bot: A real-time conversational interface.
Console Chat: A terminal-based chatbot for direct interaction.
Key Features:
AI model switching based on user input.
Persistent chat history across sessions.
Interactive bot responses with live editing.
Retry mechanism for robust API handling.
System Design
Architecture
The system comprises the following components:
Bot Interface: Handles incoming messages, processes user input, and sends responses.
Assistant Utilities: Contains AI model integration logic.
History Management: Manages conversation history for persistence.
Error Handling: Ensures graceful error recovery.
Flow Diagram
[User] [Telegram API] [Bot API Handler] [AI Processing Logic] ^ | | v [History Management] [Error Handler]
Components
- Telegram Bot API: Utilized for real-time messaging and updates.
- Golang’s Concurrency: To handle multiple user conversations seamlessly.
- History Module: Saves chat history in local files for reloadable sessions.
- Assistant Utilities: Processes user input and integrates AI logic.
Key Code Walkthrough
- Main Application Entry The main() function provides users with two modes: Telegram Bot and Console Chat. This ensures the assistant is accessible both online and offline.
func main() { fmt.Println("Choose mode: [1] Telegram Bot, [2] Console Chat") var choice int fmt.Scan(&choice) switch choice { case 1: deploy.TelegramBot() case 2: runConsoleChat() default: fmt.Println("Invalid choice.") } }
- Telegram Bot Initialization The bot uses go-telegram-bot-api for interacting with Telegram.
func NewTelegramBot() { token := os.Getenv("TELEGRAM_BOT_TOKEN") bot, err := NewBot(token) if err != nil { log.Fatal("Failed to start bot:", err) } bot.Start(context.Background()) }
- Handling User Messages
This method manages user interactions, including history loading and AI response handling.
[User] [Telegram API] [Bot API Handler] [AI Processing Logic] ^ | | v [History Management] [Error Handler]
- Persistent History Management Chat history is managed through file-based persistence for continuity between sessions.
func main() { fmt.Println("Choose mode: [1] Telegram Bot, [2] Console Chat") var choice int fmt.Scan(&choice) switch choice { case 1: deploy.TelegramBot() case 2: runConsoleChat() default: fmt.Println("Invalid choice.") } }
- AI Processing Logic The bot integrates with an AI assistant, handling retries and partial responses.
func NewTelegramBot() { token := os.Getenv("TELEGRAM_BOT_TOKEN") bot, err := NewBot(token) if err != nil { log.Fatal("Failed to start bot:", err) } bot.Start(context.Background()) }
Want a demo ?

Building a Golang Telegram Bot for Personal AI Assistance | by Mukul Saini | Dec, 2024 | Medium
Mukul Saini ・ ・
Medium

demo
Conclusion
With this bot, we leverage Golang's concurrency and efficient libraries to build a scalable and interactive AI assistant. The integration with Telegram API ensures a seamless real-time experience for users. Start building yours today and explore the power of AI-driven conversations!
The above is the detailed content of Building a Golang Telegram Bot for Personal AI Assistance. For more information, please follow other related articles on the PHP Chinese website!
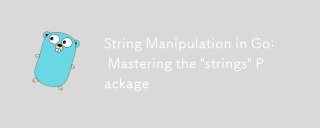
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
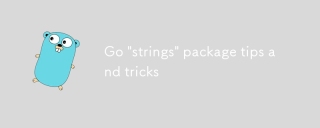
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
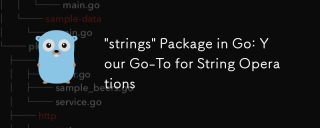
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
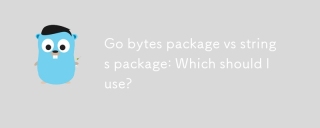
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
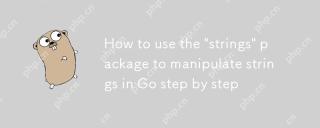
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
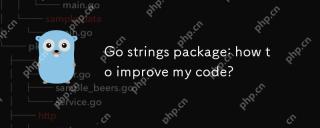
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
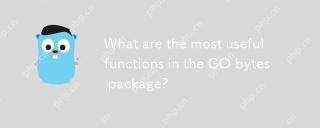
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
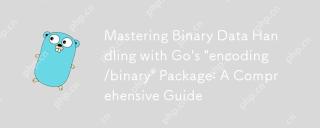
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
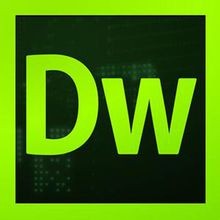
Dreamweaver CS6
Visual web development tools
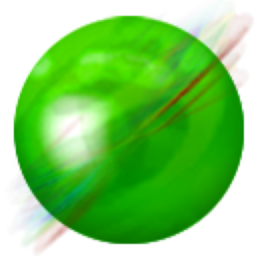
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
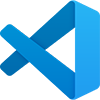
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
