


Conditional Resolving in Unity Dependency Injection
Conditional resolving allows the container to resolve and inject different implementations of an interface based on specific criteria. In this case, we aim to use Unity to conditionally resolve different authentication mechanisms based on the type of authentication used (e.g., Twitter, Facebook, etc.).
Interfaces
Define an IAuthenticate interface to represent the authentication behavior. Add an AppliesTo method to check if the provider applies to the specified provider name.
public interface IAuthenticate { bool Login(string user, string pass); bool AppliesTo(string providerName); }
Authentication Providers
Create separate classes (e.g., TwitterAuth and FacebookAuth) that implement IAuthenticate and contain the specific authentication logic. In the AppliesTo method, determine if the provider is applicable based on the provided provider name.
public class TwitterAuth : IAuthenticate { bool Login(string user, string pass) { /* Logic to connect to Twitter API */ } bool AppliesTo(string providerName) { return this.GetType().Name.Equals(providerName); } }
Strategy
Implement an IAuthenticateStrategy interface to encapsulate the conditional resolution logic. Inject an array of IAuthenticate providers and use the AppliesTo method to select the correct provider for authentication.
public interface IAuthenticateStrategy { bool Login(string providerName, string user, string pass); } public class AuthenticateStrategy : IAuthenticateStrategy { private readonly IAuthenticate[] _authenticateProviders; public AuthenticateStrategy(IAuthenticate[] authenticateProviders) => _authenticateProviders = authenticateProviders; public bool Login(string providerName, string user, string pass) { var provider = _authenticateProviders.FirstOrDefault(x => x.AppliesTo(providerName)); if (provider == null) { throw new Exception("Login provider not registered"); } return provider.Login(user, pass); } }
Unity Registration
Register the authentication providers and the strategy with Unity, specifying the provider names for conditional resolution.
unityContainer.RegisterType<iauthenticate twitterauth>("twitterAuth"); unityContainer.RegisterType<iauthenticate facebookauth>("facebookAuth"); unityContainer.RegisterType<iauthenticatestrategy authenticatestrategy>( new InjectionConstructor( new ResolvedArrayParameter<iauthenticate>( new ResolvedParameter<iauthenticate>("twitterAuth"), new ResolvedParameter<iauthenticate>("facebookAuth") ) ));</iauthenticate></iauthenticate></iauthenticate></iauthenticatestrategy></iauthenticate></iauthenticate>
Usage
In the controller, inject the IAuthenticateStrategy and use it to perform conditional authentication based on the provider name.
public AuthenticateController(IAuthenticateStrategy authenticateStrategy) { if (authenticateStrategy == null) throw new ArgumentNullException(nameof(authenticateStrategy)); _authenticateStrategy = authenticateStrategy; } public virtual ActionResult Twitter(string user, string pass) { bool success = _authenticateStrategy.Login("TwitterAuth", user, pass); /* Authenticate using Twitter */ } public virtual ActionResult Facebook(string user, string pass) { bool success = _authenticateStrategy.Login("FacebookAuth", user, pass); /* Authenticate using Facebook */ }
unity.config
Alternatively, you can perform the Unity registration in the unity.config file.
<register type="IAuthenticate" mapto="TwitterAuth" name="twitterAuth"></register> <register type="IAuthenticate" mapto="FacebookAuth" name="facebookAuth"></register> <register type="IAuthenticateStrategy" mapto="AuthenticateStrategy"></register>
The above is the detailed content of How can Unity Dependency Injection be used to conditionally resolve different authentication mechanisms based on provider type?. For more information, please follow other related articles on the PHP Chinese website!
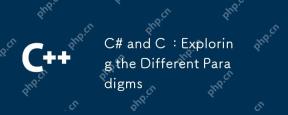
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
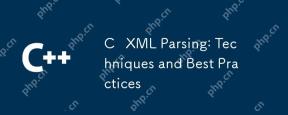
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
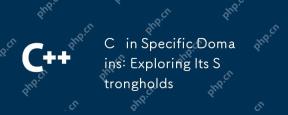
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
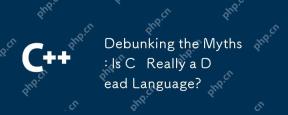
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
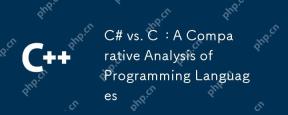
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
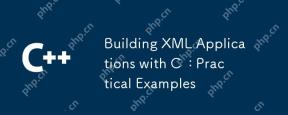
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
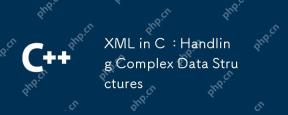
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
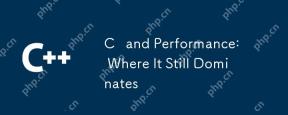
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
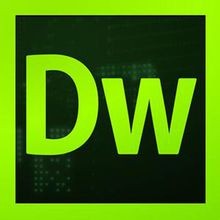
Dreamweaver CS6
Visual web development tools
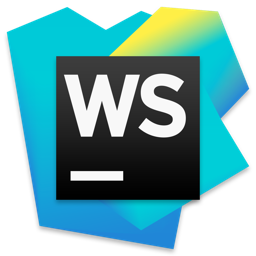
WebStorm Mac version
Useful JavaScript development tools
