


INSERT INTO or UPDATE with Two Conditions
This query involves the challenge of efficiently managing data in a table where new rows are inserted and existing rows need to be updated based on specific conditions. The table has the following schema:
ID INTEGER PRIMARY KEY AUTOINCREMENT name INTEGER values1 INTEGER values2 INTEGER dates DATE
The objective is to insert a new row when there is new data for a given 'name' and 'dates' combination. However, if a row with the same 'name' and 'dates' already exists, it should be updated instead of inserting a duplicate row.
An initial solution could involve the use of a Stored Procedure (SPROC) to check the condition and execute the appropriate action. However, as the data is being pushed from another language, this approach is not feasible.
The optimal solution lies in utilizing the INSERT INTO ... ON DUPLICATE KEY UPDATE syntax. This allows for a single statement to both insert new rows and update existing rows based on the specified conditions.
In this scenario, you can define a unique composite key (name, dates) on the table:
unique key(name,dates)
This unique key ensures that the combination of 'name' and 'dates' cannot exist in the table more than once. When executing the INSERT query, you can use the ON DUPLICATE KEY UPDATE clause to specify the action to be taken if a unique key violation occurs:
INSERT INTO myThing (name, values1, values2, dates) VALUES (777, 1, 1, '2015-07-11') ON DUPLICATE KEY UPDATE values2 = values2 + 1;
With this approach, if a row with the 'name' and 'dates' provided already exists, its values2 column will be incremented instead of creating a new row. This behavior effectively updates the existing row with the new data.
To illustrate the functionality, consider the following example:
-- Create the table with the composite unique key CREATE TABLE myThing ( id INT AUTO_INCREMENT PRIMARY KEY, name INT NOT NULL, values1 INT NOT NULL, values2 INT NOT NULL, dates DATE NOT NULL, UNIQUE KEY (name, dates) ); -- Insert a new row or update an existing row INSERT INTO myThing (name, values1, values2, dates) VALUES (777, 1, 1, '2015-07-11') ON DUPLICATE KEY UPDATE values2 = values2 + 1; -- Insert another new row or update the existing row for 'name' 777 INSERT INTO myThing (name, values1, values2, dates) VALUES (777, 1, 1, '2015-07-11') ON DUPLICATE KEY UPDATE values2 = values2 + 1; -- Insert a new row for 'name' 778 INSERT INTO myThing (name, values1, values2, dates) VALUES (778, 1, 1, '2015-07-11') ON DUPLICATE KEY UPDATE values2 = values2 + 1; -- Retrieve the results SELECT * FROM myThing;
This will produce the following output:
+----+------+---------+---------+------------+ | id | name | values1 | values2 | dates | +----+------+---------+---------+------------+ | 1 | 777 | 1 | 2 | 2015-07-11 | | 2 | 778 | 1 | 1 | 2015-07-11 | +----+------+---------+---------+------------+
As evident, the row for 'name' 777 was updated with the new data, while a new row was created for 'name' 778.
The above is the detailed content of How can I efficiently insert or update rows in a database table based on two conditions using a single SQL statement?. For more information, please follow other related articles on the PHP Chinese website!
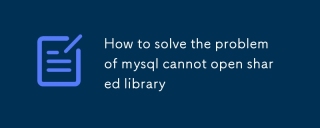
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
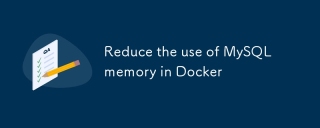
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
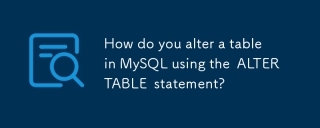
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
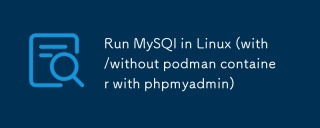
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
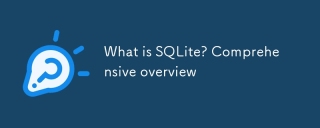
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
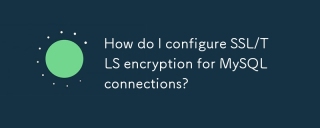
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
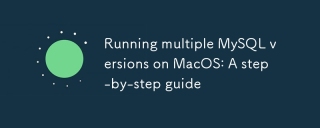
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
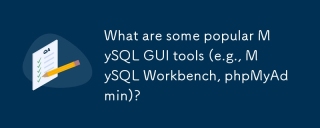
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
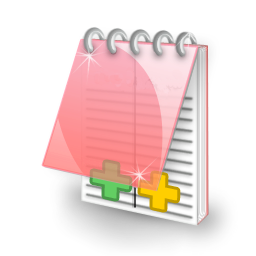
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use
