Scalable TCP/IP Server Design Pattern
When designing a scalable TCP/IP server that requires long-running connections, it's crucial to consider the most efficient network architecture. Asynchronous sockets are a recommended approach due to their ability to handle multiple clients simultaneously without consuming excessive resources.
Network Architecture
-
Server:
- Start a service with at least one thread to handle incoming connections.
- Implement asynchronous sockets using the BeginReceive and EndReceive methods.
- Create a class to manage client connections, including a list to hold references to active clients.
- Use the BeginAccept method on the server socket to listen for incoming connections and call the AcceptCallback when a connection is established.
-
Client:
- Connect to the server using a socket.
- Send and receive data through the socket asynchronously using BeginSend and BeginReceive.
Data Flow
- Data primarily flows from the server to the clients, with occasional commands from the clients.
- The server sends status data periodically to the clients.
- Received data from clients can be buffered and processed asynchronously, creating jobs on the thread pool to prevent delays in further data reception.
Example Code
using System; using System.Net; using System.Net.Sockets; namespace TcpServer { class xConnection { public byte[] buffer; public System.Net.Sockets.Socket socket; } class Server { private List<xconnection> _sockets; private System.Net.Sockets.Socket _serverSocket; private int _port; private int _backlog; public bool Start() { IPHostEntry localhost = Dns.GetHostEntry(Dns.GetHostName()); IPEndPoint serverEndPoint = new IPEndPoint(localhost.AddressList[0], _port); try { _serverSocket = new Socket(serverEndPoint.Address.AddressFamily, SocketType.Stream, ProtocolType.Tcp); _serverSocket.Bind(serverEndPoint); _serverSocket.Listen(_backlog); _serverSocket.BeginAccept(new AsyncCallback(AcceptCallback), _serverSocket); return true; } catch (Exception e) { // Handle exceptions appropriately return false; } } private void AcceptCallback(IAsyncResult result) { try { Socket serverSocket = (Socket)result.AsyncState; xConnection conn = new xConnection(); conn.socket = serverSocket.EndAccept(result); conn.buffer = new byte[_bufferSize]; lock (_sockets) { _sockets.Add(conn); } conn.socket.BeginReceive(conn.buffer, 0, conn.buffer.Length, SocketFlags.None, new AsyncCallback(ReceiveCallback), conn); _serverSocket.BeginAccept(new AsyncCallback(AcceptCallback), _serverSocket); } catch (Exception e) { // Handle exceptions appropriately } } private void Send(byte[] message, xConnection conn) { if (conn != null && conn.socket.Connected) { lock (conn.socket) { conn.socket.Send(message, message.Length, SocketFlags.None); } } } } }</xconnection>
Additional Considerations
- Consider using a reassembly protocol for handling incoming data fragments.
- Use a single BeginAccept at any one time to avoid potential bugs.
- Synchronize access to shared resources, such as the client list, to ensure thread safety.
The above is the detailed content of How to Design a Scalable TCP/IP Server with Long-Running Connections?. For more information, please follow other related articles on the PHP Chinese website!
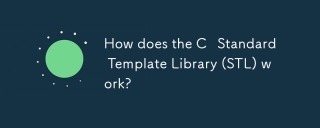
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
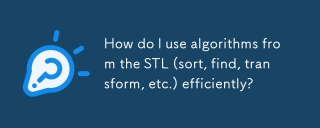
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
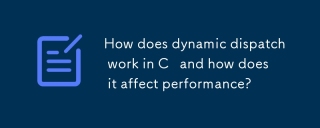
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
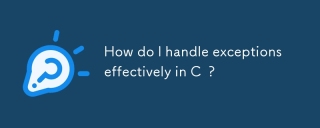
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
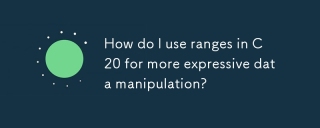
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
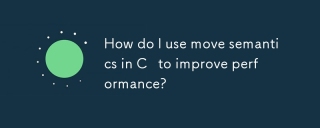
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
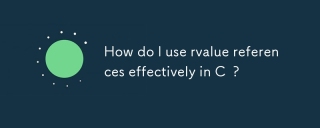
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
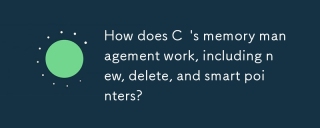
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
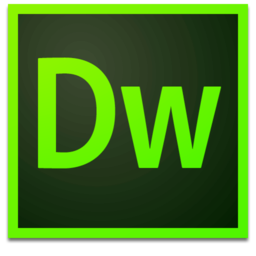
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
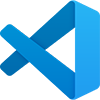
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
