


How Can I Work with Go Maps Having the Same Key Type but Different Value Types?
Extending Map Usages with Heterogeneous Value Types
Maps in Go provide a powerful data structure for managing key-value pairs. However, when working with collections of maps possessing the same key type but varying value types, the lack of built-in support can become a limitation.
Consider the following code snippet:
func useKeys(m map[string]interface{}) { //something with keys here }
The intention is to write a generic function that operates on the keys of any map with a string key type, regardless of the value type. However, attempts like the one above fail due to the type mismatch.
The fundamental challenge lies in the absence of covariance in Go's map and slice types. Unlike generic languages, Go does not support covariantly subtyping, meaning that a map with a derived key or value type is not compatible with a map with a base key or value type.
Practical Approaches
While there is no elegant solution to this problem, there are practical workarounds:
Explicit Type Handling:
One approach is to create separate functions for each specific map type. For example:
func useKeysInts(m map[string]int) { //operations on string keys and int values } func useKeysDoubles(m map[string]double) { //operations on string keys and double values }
Reflection-Based Approach:
Reflection provides a more dynamic solution, allowing you to examine and manipulate the map's structure and contents. The following function uses reflection to extract the keys from any map:
func useKeysReflect(m interface{}) { v := reflect.ValueOf(m) if v.Kind() != reflect.Map { fmt.Println("not a map!") return } keys := v.MapKeys() fmt.Println(keys) }
This approach is useful when you need to perform operations on the keys of a map of unknown or dynamically generated types.
It's worth noting that the reflection-based approach may incur some runtime overhead due to the additional processing required.
The above is the detailed content of How Can I Work with Go Maps Having the Same Key Type but Different Value Types?. For more information, please follow other related articles on the PHP Chinese website!
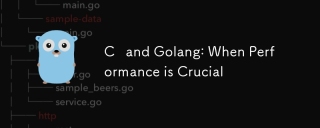
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
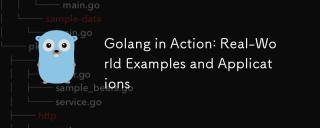
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
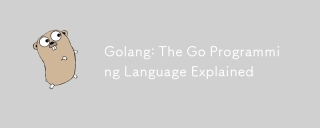
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
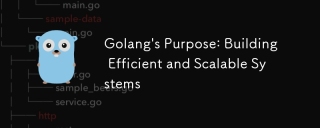
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
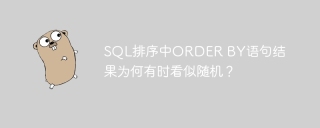
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
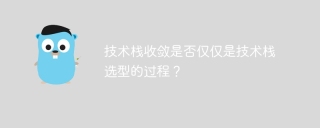
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
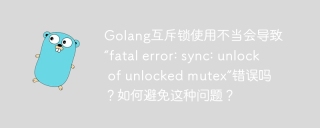
Golang ...
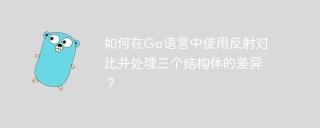
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
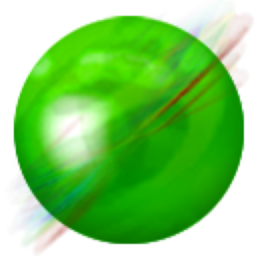
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
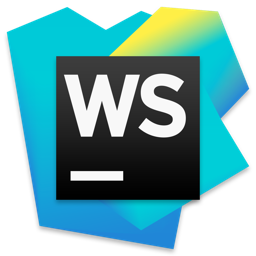
WebStorm Mac version
Useful JavaScript development tools
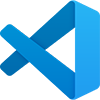
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft