


Passing Parameters by Value: Rethinking const std::string &
Herb Sutter recently questioned the conventional practice of passing std::vector and std::string objects by const reference. He suggests that in many cases, passing them by value is now preferable, as demonstrated in the following code:
std::string do_something(std::string inval) { std::string return_val; // ... do stuff ... return return_val; }
While the return value is an rvalue at the point of function return and can be moved cost-effectively, the rationale for Sutter's statement lies in cases like this:
Consider a function A that invokes function B, which in turn invokes function C. A passes a string parameter to B, which forwards it to C. A has no direct knowledge of C.
If B and C take the string by const reference, the code would appear as follows:
void B(const std::string &str) { C(str); } void C(const std::string &str) { // Process `str` without storing it. }
With this approach, passing and receiving pointers suffices, avoiding costly copying or moving.
However, if C needs to store the string:
void C(const std::string &str) { // Process `str`. m_str = str; }
This triggers a copy constructor and potential memory allocation (ignoring Short String Optimization). While C 11's move semantics aim to eliminate unnecessary copying, C taking a const reference prevents this, despite A passing a temporary string.
Conversely, if str were passed by value through all functions, relying on move semantics to transfer ownership, C could either adopt or discard the data without causing a copy operation. This approach comes with a slight performance overhead, but it eliminates memory allocations, which may be advantageous depending on the specific use case.
The above is the detailed content of Should You Pass `std::string` and `std::vector` by Value or Const Reference?. For more information, please follow other related articles on the PHP Chinese website!
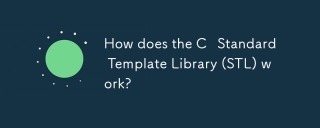
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
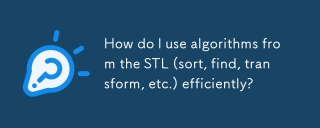
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
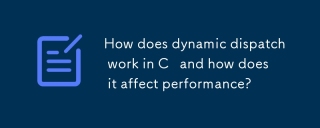
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
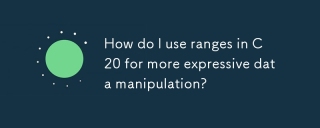
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
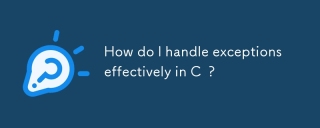
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
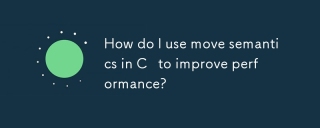
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
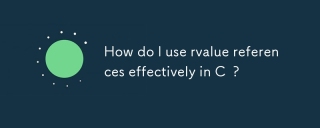
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
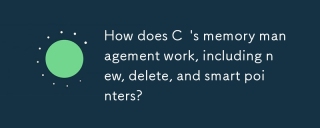
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
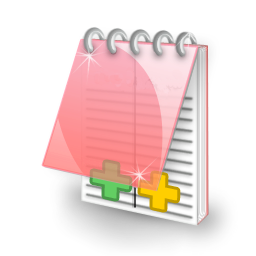
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
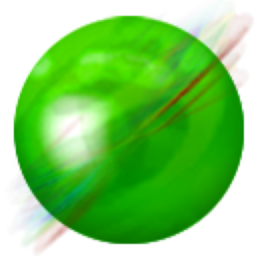
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
