


How Can I Effectively Use Unions in Go Generics for Type Safety and Flexibility?
Unions in Go Generics
When working with generics in Go, it's important to understand the concept of unions. A union is a type set used in interface constraints. Here's a breakdown of the issue you encountered:
You are creating a Difference function that returns unique elements from multiple slices. Initially, you define intOrString as an interface containing both int and string types.
However, Go requires that interface constraints only be used in type parameter lists, not as types. Instead, you should use intOrString as a constraint in the type parameters of your testDifferenceInput, testDifferenceOutput, and testDifference types:
type testDifferenceInput[T intOrString] [][]T type testDifferenceOutput[T intOrString] []T type testDifference[T intOrString] struct { input testDifferenceInput[T] output testDifferenceOutput[T] }
Another issue you faced was that the test slice contained different slice types, such as testDifference[int] and testDifference[string]. Even though the testDifference type is generic, its concrete instantiations are not interchangeable. If you need to hold different types of slices, you must either use []interface{} or separate them into distinct slices.
Finally, remember that only operations permitted by every member of the union's type set are allowed on union constraints. In the case of int | string, the permitted operations include variable declarations, conversions, comparisons, ordering, and the addition operator.
By following these guidelines, you can effectively utilize unions in your Go generic code to enhance type safety and flexibility.
The above is the detailed content of How Can I Effectively Use Unions in Go Generics for Type Safety and Flexibility?. For more information, please follow other related articles on the PHP Chinese website!
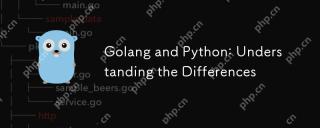
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
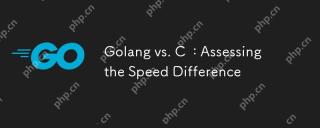
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
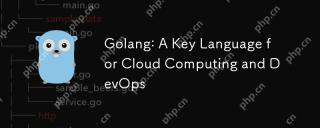
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
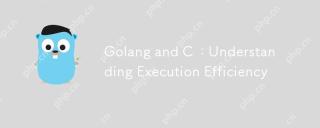
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
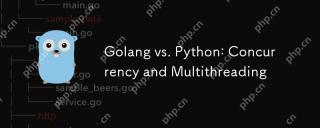
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
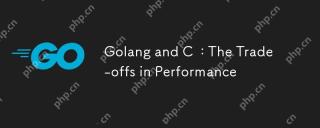
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
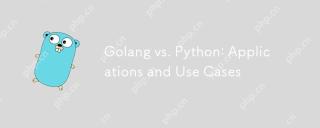
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
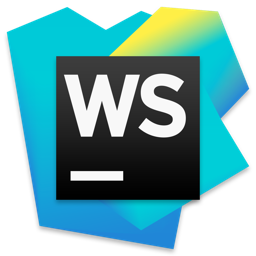
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
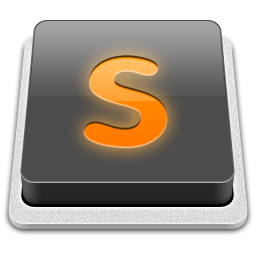
SublimeText3 Mac version
God-level code editing software (SublimeText3)