Introduction
Building real-world projects is the best way to master Go programming. Here are five advanced project ideas that will help you understand different aspects of Go and build your portfolio.
1. Distributed Task Scheduler
Project Overview
Build a distributed task scheduler similar to Airflow or Temporal but simplified. This project will help you understand distributed systems, job scheduling, and fault tolerance.
Key Features
Distributed task execution
DAG-based workflow definition
Task retry mechanisms
Web UI for monitoring
REST API for task management
Technical Implementation
// Task definition type Task struct { ID string Name string Dependencies []string Status TaskStatus Retries int MaxRetries int Handler func(ctx context.Context) error } // DAG definition type DAG struct { ID string Tasks map[string]*Task Graph *directed.Graph } // Scheduler implementation type Scheduler struct { mu sync.RWMutex dags map[string]*DAG executor *Executor store Storage } func (s *Scheduler) ScheduleDAG(ctx context.Context, dag *DAG) error { s.mu.Lock() defer s.mu.Unlock() // Validate DAG if err := dag.Validate(); err != nil { return fmt.Errorf("invalid DAG: %w", err) } // Store DAG if err := s.store.SaveDAG(ctx, dag); err != nil { return fmt.Errorf("failed to store DAG: %w", err) } // Schedule ready tasks readyTasks := dag.GetReadyTasks() for _, task := range readyTasks { s.executor.ExecuteTask(ctx, task) } return nil }
Learning Outcomes
Distributed systems design
Graph algorithms
State management
Concurrency patterns
Error handling
2. Real-time Analytics Engine
Project Overview
Create a real-time analytics engine that can process streaming data and provide instant analytics. This project will teach you about data processing, streaming, and real-time analytics.
Key Features
Real-time data ingestion
Stream processing
Aggregation pipelines
Real-time dashboards
Historical data analysis
Technical Implementation
// Stream processor type Processor struct { input chan Event output chan Metric store TimeSeriesStore } type Event struct { ID string Timestamp time.Time Type string Data map[string]interface{} } type Metric struct { Name string Value float64 Tags map[string]string Timestamp time.Time } func NewProcessor(bufferSize int) *Processor { return &Processor{ input: make(chan Event, bufferSize), output: make(chan Metric, bufferSize), store: NewTimeSeriesStore(), } } func (p *Processor) ProcessEvents(ctx context.Context) { for { select { case event := <h3> Learning Outcomes </h3>
Stream processing
Time series databases
Real-time data handling
Performance optimization
Data aggregation
3. Container Orchestration Platform
Project Overview
Build a simplified container orchestration platform similar to a basic version of Kubernetes. This will help you understand container management, networking, and system design.
Key Features
Container lifecycle management
Service discovery
Load balancing
Health checking
Resource allocation
Technical Implementation
// Container orchestrator type Orchestrator struct { nodes map[string]*Node services map[string]*Service scheduler *Scheduler } type Container struct { ID string Image string Status ContainerStatus Node *Node Resources ResourceRequirements } type Service struct { Name string Containers []*Container Replicas int LoadBalancer *LoadBalancer } func (o *Orchestrator) DeployService(ctx context.Context, spec ServiceSpec) error { service := &Service{ Name: spec.Name, Replicas: spec.Replicas, } // Schedule containers across nodes for i := 0; i <h3> Learning Outcomes </h3>
Container management
Network programming
Resource scheduling
High availability
System architecture
4. Distributed Search Engine
Project Overview
Create a distributed search engine with features like full-text search, indexing, and ranking. This project will teach you about search algorithms, distributed indexing, and information retrieval.
Key Features
Distributed indexing
Full-text search
Ranking algorithms
Query parsing
Horizontal scaling
Technical Implementation
// Task definition type Task struct { ID string Name string Dependencies []string Status TaskStatus Retries int MaxRetries int Handler func(ctx context.Context) error } // DAG definition type DAG struct { ID string Tasks map[string]*Task Graph *directed.Graph } // Scheduler implementation type Scheduler struct { mu sync.RWMutex dags map[string]*DAG executor *Executor store Storage } func (s *Scheduler) ScheduleDAG(ctx context.Context, dag *DAG) error { s.mu.Lock() defer s.mu.Unlock() // Validate DAG if err := dag.Validate(); err != nil { return fmt.Errorf("invalid DAG: %w", err) } // Store DAG if err := s.store.SaveDAG(ctx, dag); err != nil { return fmt.Errorf("failed to store DAG: %w", err) } // Schedule ready tasks readyTasks := dag.GetReadyTasks() for _, task := range readyTasks { s.executor.ExecuteTask(ctx, task) } return nil }
Learning Outcomes
Information retrieval
Distributed systems
Text processing
Ranking algorithms
Query optimization
5. Distributed Key-Value Store
Project Overview
Build a distributed key-value store with features like replication, partitioning, and consistency. This project will help you understand distributed databases and consensus algorithms.
Key Features
Distributed storage
Replication
Partitioning
Consistency protocols
Failure handling
Technical Implementation
// Stream processor type Processor struct { input chan Event output chan Metric store TimeSeriesStore } type Event struct { ID string Timestamp time.Time Type string Data map[string]interface{} } type Metric struct { Name string Value float64 Tags map[string]string Timestamp time.Time } func NewProcessor(bufferSize int) *Processor { return &Processor{ input: make(chan Event, bufferSize), output: make(chan Metric, bufferSize), store: NewTimeSeriesStore(), } } func (p *Processor) ProcessEvents(ctx context.Context) { for { select { case event := <h3> Learning Outcomes </h3>
Distributed consensus
Data replication
Partition tolerance
Consistency patterns
Failure recovery
Conclusion
These projects cover various aspects of advanced Go programming and distributed systems. Each project will help you master different aspects of Go and build practical experience with real-world applications.
Tips for Implementation
Start with a minimal viable version
Add features incrementally
Write comprehensive tests
Document your code
Consider scalability from the start
Share your project implementations and experiences in the comments below!
Tags: #golang #programming #projects #distributed-systems #backend
The above is the detailed content of dvanced Golang Projects to Build Your Expertise. For more information, please follow other related articles on the PHP Chinese website!
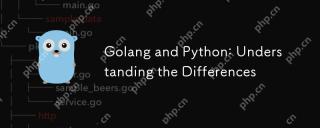
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
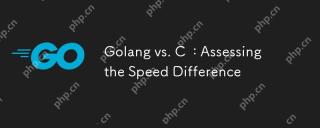
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
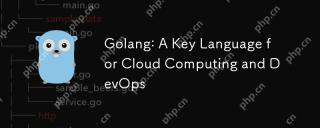
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
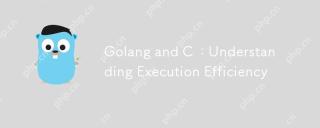
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
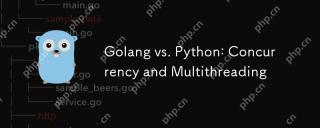
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
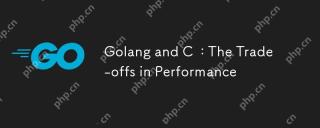
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
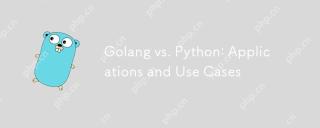
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
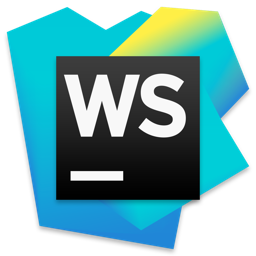
WebStorm Mac version
Useful JavaScript development tools
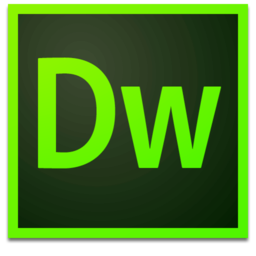
Dreamweaver Mac version
Visual web development tools
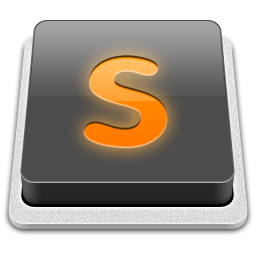
SublimeText3 Mac version
God-level code editing software (SublimeText3)