How can I preserve image quality when downsizing large images significantly?
Large Scale Image Downsizing with Quality Preservation
Downsizing images excessively can present quality challenges. To tackle this, divide and conquer is an effective method. Here's how:
1. Original Image:
2. Incremental Downsizing:
Instead of resizing from 300x300 to 60x60 in one step, scale gradually:
- Resize to 150x150 (1/2 of original dimension)
- Resize to 75x75 (1/2 of 150x150)
- Resize to 37x38 (1/2 of 75x75)
- Resize to 60x60 (1/2 of 37x38)
Each step reduces the image dimension by half.
3. Result:
4. Comparison (One Step vs. Divide and Conquer):
5. Java Implementation:
public class DivideAndConquerImageResizer { public static main(String[] args) { // Load the original image BufferedImage original = ImageIO.read(...); // Create a scaled image BufferedImage scaled = null; Dimension currentSize = new Dimension(original.getWidth(), original.getHeight()); Dimension desiredSize = new Dimension(60, 60); while (currentSize.getWidth() > desiredSize.getWidth() || currentSize.getHeight() > desiredSize.getHeight()) { // Halve the current size currentSize.width = (int) Math.ceil(currentSize.width / 2.0); currentSize.height = (int) Math.ceil(currentSize.height / 2.0); // Resize the image to the current size scaled = getScaledInstanceToFit(original, currentSize); } // Save the scaled image ImageIO.write(scaled, "jpg", ...); } public static BufferedImage getScaledInstanceToFit(BufferedImage img, Dimension size) { float scaleFactor = getScaleFactorToFit(img, size); return getScaledInstance(img, scaleFactor); } public static float getScaleFactorToFit(BufferedImage img, Dimension size) { float scale = 1f; if (img != null) { int imageWidth = img.getWidth(); int imageHeight = img.getHeight(); scale = getScaleFactorToFit(new Dimension(imageWidth, imageHeight), size); } return scale; } public static float getScaleFactorToFit(Dimension original, Dimension toFit) { float scale = 1f; if (original != null && toFit != null) { float dScaleWidth = getScaleFactor(original.width, toFit.width); float dScaleHeight = getScaleFactor(original.height, toFit.height); scale = Math.min(dScaleHeight, dScaleWidth); } return scale; } public static float getScaleFactor(int iMasterSize, int iTargetSize) { float scale = 1; if (iMasterSize > iTargetSize) { scale = (float) iTargetSize / (float) iMasterSize; } else { scale = (float) iTargetSize / (float) iMasterSize; } return scale; } public static BufferedImage getScaledInstance(BufferedImage img, double dScaleFactor) { BufferedImage imgBuffer = null; imgBuffer = getScaledInstance(img, dScaleFactor, RenderingHints.VALUE_INTERPOLATION_BILINEAR, true); return imgBuffer; } }
This approach yields noticeably improved image quality, especially when downsizing significantly.
The above is the detailed content of How can I preserve image quality when downsizing large images significantly?. For more information, please follow other related articles on the PHP Chinese website!
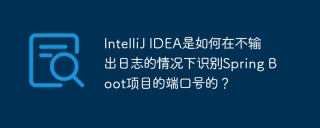
Start Spring using IntelliJIDEAUltimate version...
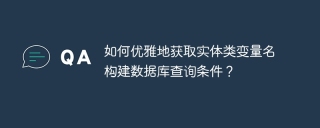
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
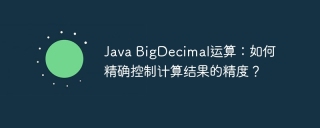
Java...
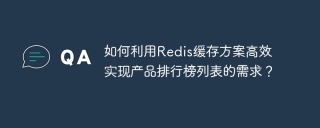
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
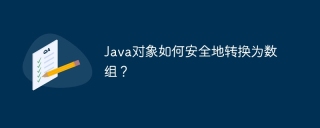
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
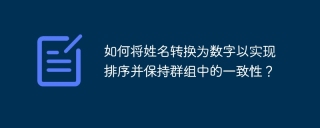
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
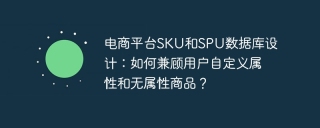
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
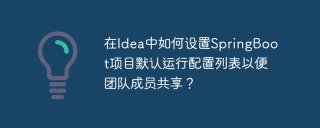
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
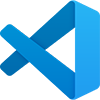
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
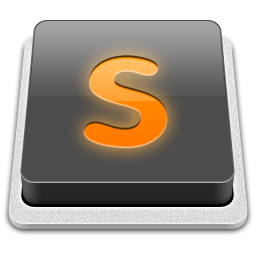
SublimeText3 Mac version
God-level code editing software (SublimeText3)