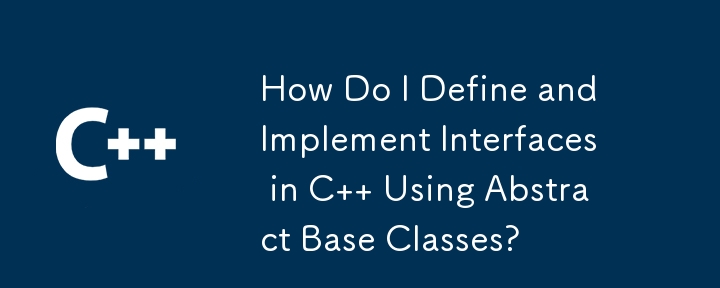
Declaring Interfaces in C
Question:
How can I define a class representing an interface in C ? Is this achieved through an abstract base class?
Answer:
Yes, an interface in C can be defined as an abstract base class. To create an interface:
-
Declare a pure abstract class: This class should contain only pure virtual methods, which have no implementation. The methods serve as placeholders and force derived classes to implement them.
class IDemo {
public:
virtual void OverrideMe() = 0;
};
-
Derived classes: Classes that inherit from the interface must implement all pure virtual methods. They provide the concrete implementation of the interface.
class Child : public IDemo {
public:
virtual void OverrideMe() {
// Implement the method here
}
};
-
Virtual destructor (optional): Consider adding a virtual destructor to the interface. This allows safe pointer ownership transfer without exposing the concrete derived class.
class IDemo {
public:
virtual ~IDemo() {}
virtual void OverrideMe() = 0;
};
Additional Notes:
- Unlike in other languages, C interfaces do not support multiple inheritance.
- The destructor does not require a body since the interface has no concrete members.
- Some compilers may have issues with empty virtual destructors, so it's recommended not to include a body.
- Using virtual and inline functions together for the destructor is acceptable.
The above is the detailed content of How Do I Define and Implement Interfaces in C Using Abstract Base Classes?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn