


How Does Java Garbage Collection Handle Circular References to Prevent Memory Leaks?
How Does Java Garbage Collection Handle Circular References?
In Java, garbage collection (GC) removes unused objects from memory. However, circular references, where objects reference each other, can prevent GC from functioning correctly.
Consider the following example:
class Node { public Object value; public Node next; public Node(Object o, Node n) { value = o; next = n; } } //...some code { Node a = new Node("a", null), b = new Node("b", a), c = new Node("c", b); a.next = c; } //end of scope //...other code
In this example, a, b, and c form a cycle of references. As a result, the GC cannot reclaim these objects because there is always a reference leading back to them.
How does Java GC deal with this?
Java's GC: Unreachable Objects
Java GC identifies objects as garbage only if they are unreachable from any GC root. GC roots include global variables, static variables, thread stacks, and objects pointed to by other reachable objects.
In our example, since a, b, and c are not referenced by any GC roots, they are considered unreachable and thus garbage. Despite forming a cycle, GC will reclaim these objects.
Implications for Memory Management
Circular references do not inherently cause memory leaks in Java. As long as the objects in the circular reference are unreachable from any GC root, they will still be collected. However, it's important to be aware of circular references if they can lead to unexpected behavior or performance issues.
The above is the detailed content of How Does Java Garbage Collection Handle Circular References to Prevent Memory Leaks?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
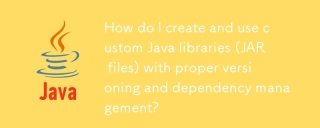
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
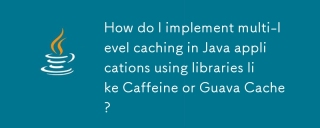
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
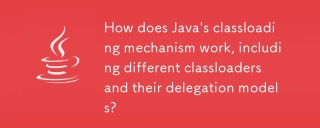
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
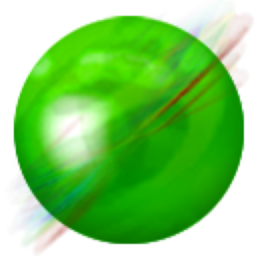
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
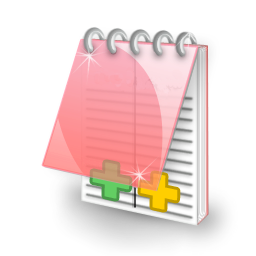
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment