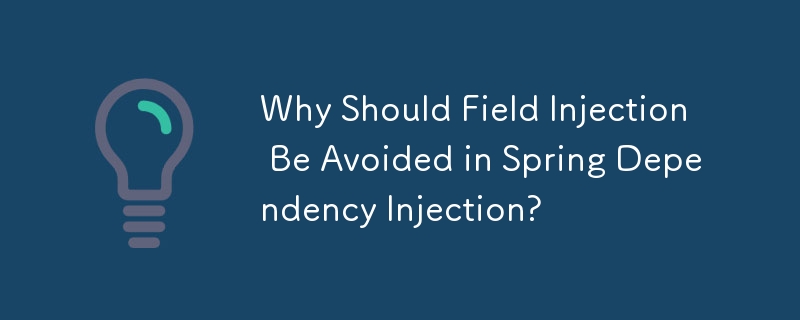
Field Injection and Its Detriments
Field injection, whereby beans are injected via @Autowired annotations on fields, is often discouraged. To delineate this, consider the following:
@Component
public class MyComponent {
@Autowired
private Cart cart;
}
Alternatively, constructor injection employs the following approach:
@Component
public class MyComponent {
private final Cart cart;
@Autowired
public MyComponent(Cart cart){
this.cart = cart;
}
}
Injection Techniques
There are three primary dependency injection methods:
-
Constructor injection: Injected through constructors.
-
Method injection: Injected through setters or other methods.
-
Field injection: Injected directly into fields using reflection.
Field injection, as seen in the first example, corresponds to the third option.
Injection Guidelines
Spring advocates the following injection guidelines:
-
Mandatory dependencies: Utilize constructor injection.
-
Optional or changeable dependencies: Employ setter injection.
-
Avoid field injection: Use it only in exceptional scenarios.
Drawbacks of Field Injection
Field injection is discouraged for several reasons:
-
Immutable objects: Constructor injection facilitates the creation of immutable objects, which field injection does not offer.
-
Tight coupling: Field injection tightly couples classes with the DI container, hindering their independent usage.
-
Instantiation challenges: Without reflection and the DI container, classes cannot be instantiated, hindering unit testing capabilities.
-
Hidden dependencies: Field injection conceals dependencies, making it difficult to discern the class's reliance on external components.
-
Multiple dependencies: Field injection enables the unfettered accumulation of dependencies.
-
Responsibility violation: Classes that handle multiple responsibilities often exhibit excessive dependencies, potentially violating the Single Responsibility Principle.
Conclusion
Constructor and setter injection should be prioritized based on requirements. Field injection should generally be avoided due to its shortcomings, with convenience as its sole advantage.
The above is the detailed content of Why Should Field Injection Be Avoided in Spring Dependency Injection?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn