Generating All Set Partitions
One of the fundamental problems in combinatorial mathematics is finding all partitions of a given set. A set partition divides the set into non-empty disjoint subsets, referred to as blocks or parts.
In this problem, we seek a method to enumerate all partitions of a set with distinct elements. Consider the set {1, 2, 3}. Its partitions are:
- {{1}, {2}, {3}}
- {{1, 2}, {3}}
- {{1, 3}, {2}}
- {{1}, {2, 3}}
- {{1, 2, 3}}
Partitioning Algorithm
The task can be broken down into two subproblems: partitioning into two parts and partitioning a part into multiple parts.
Two-Part Partitioning
For an n-element set, all two-part partitions can be generated by representing each element as a bit in an n-bit pattern. A 0 bit indicates placement in the first part, and a 1 bit indicates placement in the second part. To avoid duplicate results when swapping parts, we always assign the first element to the first part. This leaves (2^(n-1))-1 unique two-part patterns.
Recursive Partitioning
With the two-part partitioning technique in place, we can recursively construct all partitions.
- Start with an empty fixed part and the original set as the suffix.
- Generate two-part partitions of the suffix.
- For each suffix partition, recursively partition its second part into multiple parts.
- Combine the fixed parts with the recursive partitions to obtain all partitions containing the fixed parts.
- Repeat step 4 until all elements are partitioned.
C# Implementation
The following C# implementation employs the recursive partitioning algorithm:
using System; using System.Collections.Generic; using System.Linq; namespace PartitionTest { public static class Partitioning { public static IEnumerable<t> GetAllPartitions<t>(T[] elements) { return GetAllPartitions(new T[][]{}, elements); } private static IEnumerable<t> GetAllPartitions<t>(T[][] fixedParts, T[] suffixElements) { // Trivial partition: fixed parts followed by all suffix elements as a single block yield return fixedParts.Concat(new[] { suffixElements }).ToArray(); // Two-group-partitions of suffix elements and their recursive sub-partitions var suffixPartitions = GetTuplePartitions(suffixElements); foreach (Tuple<t t> suffixPartition in suffixPartitions) { var subPartitions = GetAllPartitions( fixedParts.Concat(new[] { suffixPartition.Item1 }).ToArray(), suffixPartition.Item2); foreach (var subPartition in subPartitions) { yield return subPartition; } } } private static IEnumerable<tuple t>> GetTuplePartitions<t>(T[] elements) { if (elements.Length [] resultSets = { new List<t> { elements[0] }, new List<t>() }; for (int index = 1; index > (index - 1)) & 1].Add(elements[index]); } yield return Tuple.Create(resultSets[0].ToArray(), resultSets[1].ToArray()); } } } }</t></t></t></tuple></t></t></t></t></t>
The above is the detailed content of How Can We Generate All Set Partitions of a Given Set?. For more information, please follow other related articles on the PHP Chinese website!
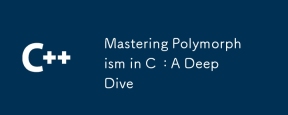
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
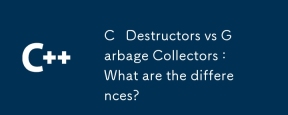
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
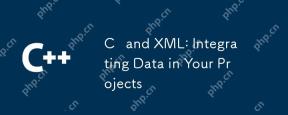
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
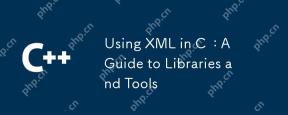
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
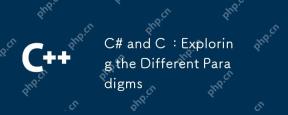
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
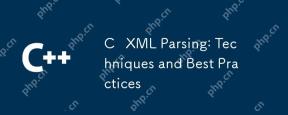
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
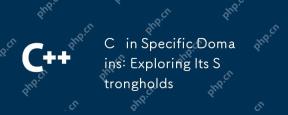
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
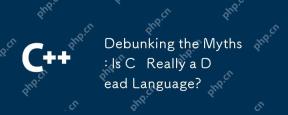
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
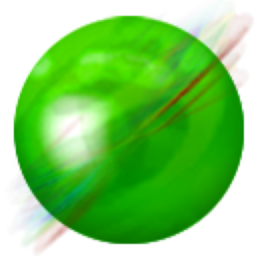
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
