3203. Find Minimum Diameter After Merging Two Trees
Difficulty: Hard
Topics: Tree, Depth-First Search, Breadth-First Search, Graph
There exist two undirected trees with n and m nodes, numbered from 0 to n - 1 and from 0 to m - 1, respectively. You are given two 2D integer arrays edges1 and edges2 of lengths n - 1 and m - 1, respectively, where edges1[i] = [ai, bi] indicates that there is an edge between nodes ai and bi in the first tree and edges2[i] = [ui, vi] indicates that there is an edge between nodes ui and vi in the second tree.
You must connect one node from the first tree with another node from the second tree with an edge.
Return the minimum possible diameter of the resulting tree.
The diameter of a tree is the length of the longest path between any two nodes in the tree.
Example 1:
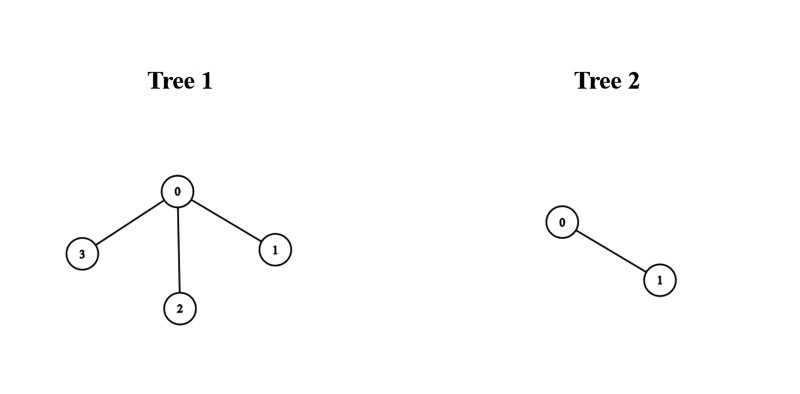
-
Input: edges1 = [[0,1],[0,2],[0,3]], edges2 = [[0,1]]
-
Output: 3
-
Explanation: We can obtain a tree of diameter 3 by connecting node 0 from the first tree with any node from the second tree.
Example 2:
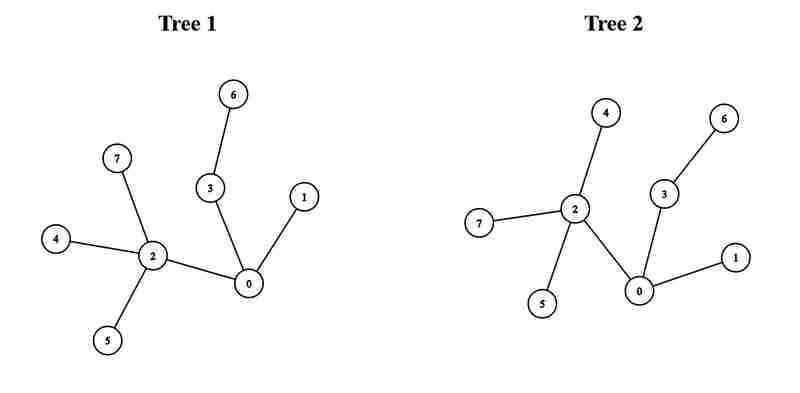
-
Input: edges1 = [[0,1],[0,2],[0,3],[2,4],[2,5],[3,6],[2,7]], edges2 = [[0,1],[0,2],[0,3],[2,4],[2,5],[3,6],[2,7]]
-
Output: 5
-
Explanation: We can obtain a tree of diameter 5 by connecting node 0 from the first tree with node 0 from the second tree.
Constraints:
- 1 <= n, m <= 105
- edges1.length == n - 1
- edges2.length == m - 1
- edges1[i].length == edges2[i].length == 2
- edges1[i] = [ai, bi]
- 0 <= ai, bi < n
- edges2[i] = [ui, vi]
- 0 <= ui, vi < m
- The input is generated such that edges1 and edges2 represent valid trees.
Hint:
- Suppose that we connected node a in tree1 with node b in tree2. The diameter length of the resulting tree will be the largest of the following 3 values:
- The diameter of tree 1.
- The diameter of tree 2.
- The length of the longest path that starts at node a and that is completely within Tree 1 The length of the longest path that starts at node b and that is completely within Tree 2 1.
- The added one in the third value is due to the additional edge that we have added between trees 1 and 2.
- Values 1 and 2 are constant regardless of our choice of a and b. Therefore, we need to pick a and b in such a way that minimizes value 3.
- If we pick a and b optimally, they will be in the diameters of Tree 1 and Tree 2, respectively. Exactly which nodes of the diameter should we pick?
-
a is the center of the diameter of tree 1, and b is the center of the diameter of tree 2.
Solution:
We need to approach it step-by-step with a focus on understanding how to calculate the diameter of a tree and how connecting the two trees influences the overall diameter.
Steps to solve:
-
Find the diameter of each tree:
- The diameter of a tree is the longest path between any two nodes. To find it, we can use the following two-step process:
- Perform a BFS (or DFS) from an arbitrary node to find the farthest node (let's call this node A).
- Perform another BFS (or DFS) starting from A to find the farthest node from A (let's call this node B), and the distance from A to B will be the diameter of the tree.
-
Determine the optimal nodes to connect:
- From the hint in the problem, the best way to minimize the additional diameter when connecting two trees is to connect the centers of the diameters of both trees. This will minimize the longest path caused by the new edge.
- The optimal node in a tree's diameter is typically the "center", which can be found by performing a BFS from the endpoints of the diameter and finding the middle of the longest path.
-
Minimize the total diameter:
- Once we find the centers of both trees, the new diameter is the maximum of:
- The diameter of Tree 1.
- The diameter of Tree 2.
- The sum of the longest path within Tree 1, the longest path within Tree 2, and 1 for the new connecting edge.
Let's implement this solution in PHP: 3203. Find Minimum Diameter After Merging Two Trees
Explanation:
BFS Helper Function: The bfs function calculates the farthest node from a given starting node and returns the distance array and the farthest node found. This is essential for calculating the diameter of the tree.
Get Diameter and Center: The getDiameterAndCenter function finds the diameter of a tree and its center. The center of the tree is crucial for minimizing the new tree's diameter when merging two trees.
-
Main Solution:
- We first build adjacency lists for both trees.
- We calculate the diameter and center for both trees.
- We perform BFS from the centers of both trees to get the longest paths within each tree.
- Finally, we calculate the maximum of the three values to get the minimum diameter of the merged tree.
Time Complexity:
- Constructing the adjacency list: O(n m)
- BFS traversal: O(n m)
- Overall time complexity is O(n m), which is efficient for the input size limit of 105.
This approach ensures we find the minimum possible diameter when merging the two trees.
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
The above is the detailed content of Find Minimum Diameter After Merging Two Trees. For more information, please follow other related articles on the PHP Chinese website!