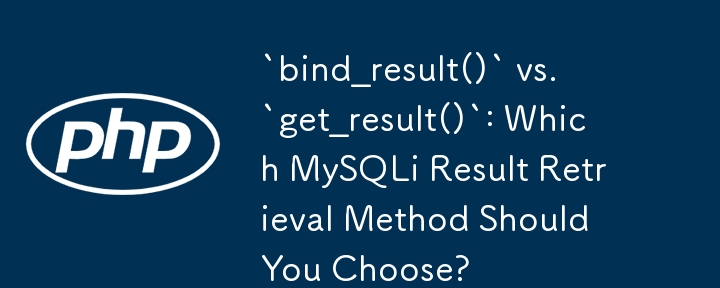
Understanding the Distinction Between bind_result() and get_result()
When querying a database using prepared statements, two primary methods for retrieving results exist: bind_result() and get_result(). Each method serves a specific purpose, and there are pros and cons to using one over the other.
bind_result()
Purpose:
- Binds specific result columns to variables for efficient retrieval.
- Useful when you know the specific columns you need and want to access them individually.
Example:
$query = "SELECT id, first_name, last_name FROM table WHERE id = ?";
$stmt = $mysqli->prepare($query);
$stmt->bind_param('i', $id);
$stmt->execute();
$stmt->bind_result($id, $first_name, $last_name);
$stmt->fetch();
Pros:
- Works with older PHP versions.
- Returns separate variables for each column, allowing for easy access.
Cons:
- Requires manual listing of all variables.
- Can become cumbersome when working with large or dynamically changing result sets.
- Code must be updated if the table structure changes.
get_result()
Purpose:
- Fetches the entire result set as an associative/enumerated array or object.
- Suitable when you need to access all columns or iterate through the results.
Example:
$query = "SELECT * FROM table WHERE id = ?";
$stmt = $mysqli->prepare($query);
$stmt->bind_param('i', $id);
$stmt->execute();
$result = $stmt->get_result();
$row = $result->fetch_assoc();
Pros:
- Returns an array or object with all data, eliminating the need for manual variable binding.
- Allows for bulk retrieval using fetch_all().
Cons:
- Requires the MySQL native driver (mysqlnd).
Limitations and Differences
-
Column Matching: bind_result() requires the explicit listing of columns in the query, while get_result() automatically matches columns to array keys.
-
Error Handling: bind_result() does not provide immediate access to error messages, while get_result() raises an exception on errors.
-
Performance: In some cases, bind_result() may be more efficient, particularly for small result sets.
In summary, bind_result() is a lightweight method for retrieving specific columns, while get_result() is a more versatile option that simplifies result processing. The choice between the two depends on the specific requirements of your application.
The above is the detailed content of `bind_result()` vs. `get_result()`: Which MySQLi Result Retrieval Method Should You Choose?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn