Limiting JTextField Input to Integers
The task of restricting JTextField input to only accept positive integers is a frequently encountered need, yet implementing this restriction can pose challenges. Using a KeyListener for this purpose, as initially attempted, has several drawbacks.
Drawbacks of Using a KeyListener:
- Inability to capture all input: A KeyListener only detects keystrokes, but it won't handle data entered via copy and paste, which can bypass the restriction.
- Low-level control: KeyListeners operate at a low abstraction level, making them less suited for Swing applications.
The Solution: DocumentFilter
A better approach is to utilize a DocumentFilter. This Swing component allows you to filter the content of a text component, providing precise control over what can be entered.
How it Works:
A DocumentFilter allows you to inspect any incoming changes to the text component's content. By checking whether the modified string represents a valid integer, you can either accept or reject the change.
Example Implementation:
The following code snippet demonstrates how to implement a DocumentFilter that restricts input to integers:
import javax.swing.text.DocumentFilter; import javax.swing.text.BadLocationException; public class MyIntFilter extends DocumentFilter { @Override public void insertString(FilterBypass fb, int offset, String string, AttributeSet attr) throws BadLocationException { Document doc = fb.getDocument(); StringBuilder sb = new StringBuilder(); sb.append(doc.getText(0, doc.getLength())); sb.insert(offset, string); if (test(sb.toString())) { super.insertString(fb, offset, string, attr); } else { // Handle invalid input, e.g., display an error message } } private boolean test(String text) { try { Integer.parseInt(text); return true; } catch (NumberFormatException e) { return false; } } }
By attaching this DocumentFilter to your JTextField, you can ensure that only valid integers are allowed as input.
The above is the detailed content of How Can I Restrict a JTextField to Accept Only Positive Integers?. For more information, please follow other related articles on the PHP Chinese website!
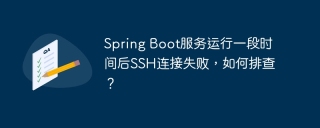
The troubleshooting idea of SSH connection failure after SpringBoot service has been running for a period of time has recently encountered a problem: a Spring...
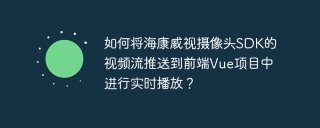
How to push video streams from Hikvision camera SDK to front-end Vue project? During the development process, you often encounter videos that need to be captured by the camera to be circulated...
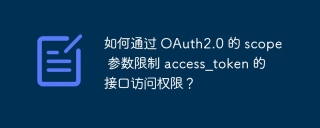
How to use access_token of OAuth2.0 to restrict interface access permissions How to ensure access_token when authorizing using OAuth2.0...
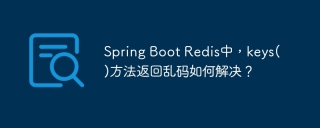
SpringBootRedis gets the key garbled problem analysis using Spring...
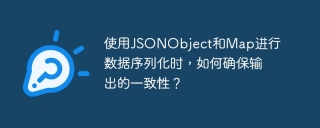
How to ensure consistency when using JSONObject and Map for data serialization? When processing JSON data, we may encounter using different methods for sequences...
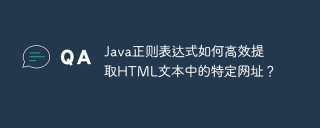
Tips for extracting specific text by Java regular expressions In Java development, it is often necessary to extract the parts we are interested in from a large amount of text data. Regular...
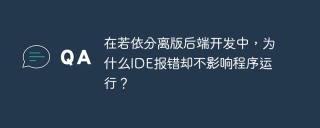
Regarding the issue of obtaining front-end data from the Ruoyi version backend When using the Ruoyi version development project, you often encounter the problem of obtaining data from the front-end and entering the back-end...
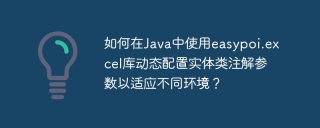
How to dynamically configure entity class annotation parameters in Java? In Java development, we often encounter the need to dynamically configure certain parameters according to different environments...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
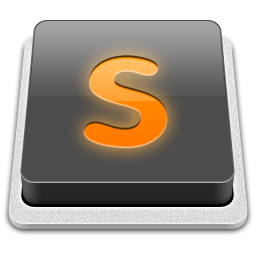
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.