Renaming Files in Java: A Comprehensive Guide
In the realm of Java file handling, one common task is renaming a file. This seemingly straightforward operation can bring forth various scenarios, each requiring a tailored solution.
Renaming a File (non-existent)
To rename a file that does not currently exist, Java provides the renameTo method. Simply specify the old and new file names as parameters, and if successful, the file will be renamed.
Renaming a File (existing)
If the destination file already exists, the renameTo operation will fail with an IOException. To handle this, you can either overwrite the existing file or append the contents of the source file to the existing file.
Appending Contents to an Existing File
To append the contents of the source file to the existing file, you can use the following code:
java.io.FileWriter out = new java.io.FileWriter(file2, true /*append=yes*/);
This code opens a file writer in append mode, ensuring that any data written to it will be added to the end of the existing file.
Complete Example
Combining the above concepts, here's a comprehensive example of how to rename a file and handle the existing file scenario:
try { // Rename file (or directory) boolean success = file.renameTo(file2); if (!success) { // File exists, handle scenario if (overwriteExisting) { // Overwrite existing file with new contents java.io.FileWriter out = new java.io.FileWriter(file2); out.write(newContents); out.close(); } else { // Append new contents to existing file java.io.FileWriter out = new java.io.FileWriter(file2, true /*append=yes*/); out.write(newContents); out.close(); } } } catch (IOException e) { // Handle IOException }
The above is the detailed content of How to Rename Files in Java: Handling Existing Files and Overwriting?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
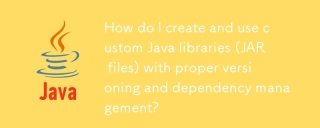
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
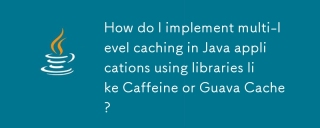
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
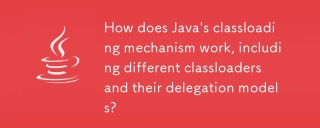
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
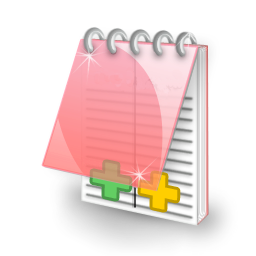
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
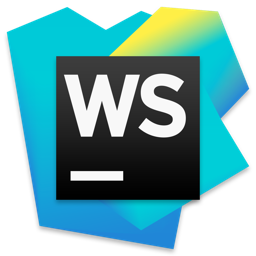
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment