Is Reading Values from Different Threads Safe?
In Go, safe concurrent access to values requires synchronization when multiple goroutines are involved, especially when at least one of them performs writes. In the given scenario, it's necessary to implement synchronization to safeguard against undefined behavior.
Recommended Approach: Utilizing Mutexes
Channels, although an option for message passing, may introduce unnecessary complexity due to the required synchronization for message retrieval in the main thread. Instead, it's recommended to employ read-write mutexes (sync.RWMutex). These locks protect data by enforcing lock acquisition for modify operations (workers) and read-only access for status retrievals (main thread).
Example Implementation
type Worker struct { iterMu sync.RWMutex iter int } func (w *Worker) Iter() int { w.iterMu.RLock() defer w.iterMu.RUnlock() return w.iter } func (w *Worker) setIter(n int) { w.iterMu.Lock() w.iter = n w.iterMu.Unlock() }
This approach ensures that workers modifying the iteration count acquire write locks, while the main thread reading the count acquires read locks.
Alternative: Atomic Operations
The sync/atomic package provides atomic operations that enable synchronization-free modification of specific data types, such as integers. It ensures integrity by restricting read and modify operations to its atomic functions.
type Worker struct { iter int64 } func (w *Worker) Iter() int64 { return atomic.LoadInt64(&w.iter) } func (w *Worker) setIter(n int64) { atomic.StoreInt64(&w.iter, n) } func (w *Worker) incIter() { atomic.AddInt64(&w.iter, 1) }
This approach offers a simpler synchronization mechanism, but note that it's only applicable for specific data types.
By adopting one of these methods, you can safely read values from workers in different threads, guaranteeing data integrity and avoiding undefined behavior.
The above is the detailed content of How to Safely Read Values from Different Goroutines in Go?. For more information, please follow other related articles on the PHP Chinese website!
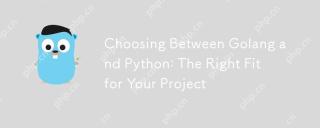
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
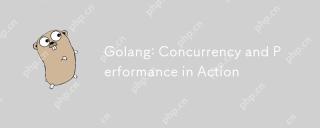
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
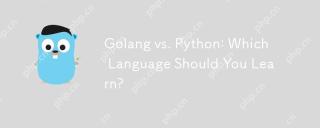
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
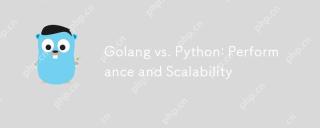
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
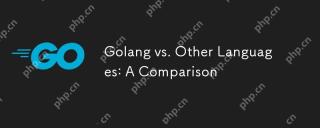
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
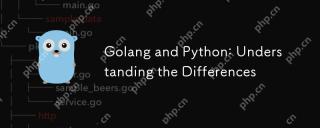
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
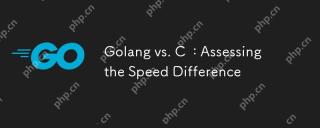
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
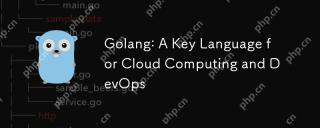
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
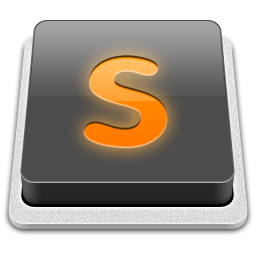
SublimeText3 Mac version
God-level code editing software (SublimeText3)