


Why Does My Image Jump When Zooming from the Mouse Cursor Using C# Transformations?
Zooming an Image from the Mouse Cursor Using Transformations
In this scenario, an attempt is made to zoom (scale) an Image from the mouse location using transformations in the Paint event to translate the bitmap origin to the mouse location and then scale the Image and translate its origin back.
Issue:
- The Image jumps and fails to scale from the relocated origin when translating the mouse location.
- Rotate, scale, and pan function correctly without translating to the mouse location.
Platform:
- .Net 4.7.2
- Visual Studio in Windows 10 1909 (18363.778)
Relevant Code Blocks:
private void trackBar1_Scroll(object sender, EventArgs e) { // Get rotation angle ang = trackBar1.Value; pnl1.Invalidate(); } private void pnl1_MouseWheel(object sender, MouseEventArgs e) { // Get mouse location mouse = e.location; // Get new scale (zoom) factor zoom = (float)(e.Delta > 0 ? zoom * 1.05 : zoom / 1.05); pnl1.Invalidate(); } private void pnl1_MouseDown(object sender, MouseEventArgs e) { if (e.Button != MouseButtons.Left) return; pan = true; mouX = e.X; mouY = e.Y; oldX = imgX; oldY = imgY; } private void pnl1_MouseMove(object sender, MouseEventArgs e) { if (e.Button != MouseButtons.Left || !pan) return; // Coordinates of panned image imgX = oldX + e.X - mouX; imgY = oldY + e.Y - mouY; pnl1.Invalidate(); } private void pnl1_MouseUp(object sender, MouseEventArgs e) { pan = false; } private void pnl1_Paint(object sender, PaintEventArgs e) { // Apply rotation angle @ center of bitmap e.Graphics.TranslateTransform(img.Width / 2, img.Height / 2); e.Graphics.RotateTransform(ang); e.Graphics.TranslateTransform(-img.Width / 2, -img.Height / 2); // Apply scaling factor - focused @ mouse location e.Graphics.TranslateTransform(mouse.X, mouse.Y, MatrixOrder.Append); e.Graphics.ScaleTransform(zoom, zoom, MatrixOrder.Append); e.Graphics.TranslateTransform(-mouse.X, -mouse.Y, MatrixOrder.Append); // Apply drag (pan) location e.Graphics.TranslateTransform(imgX, imgY, MatrixOrder.Append); // Draw "bmp" @ location e.Graphics.DrawImage(img, 0, 0); }
Possible Solutions:
To address this issue and achieve smooth zooming from the mouse location, consider the following suggestions and tricks:
1. Divide and Conquer: Break down the different graphics effects and transformations into separate, specialized methods that perform specific tasks. Then, design these methods to work together seamlessly when needed.
2. Keep It Simple: When applying multiple graphic transformations, the order in which Matrices are stacked can lead to confusion and unexpected results. It's more straightforward to calculate certain transformations (translation and scaling, primarily) beforehand and let GDI handle the rendering of pre-processed objects and shapes.
3. Use the Right Tools: A Panel as a "canvas" is not recommended for scenarios like this. It lacks double buffering, although it can be enabled. However, a PictureBox (or a non-System flat Label) offers double-buffering out of the box and is designed for drawing rather than containing child Controls.
4. Implement Zoom Modes: Instead of blindly scaling from the mouse location, provide different methods to control the zoom behavior. Implement zoom modes such as ImageLocation, CenterCanvas, CenterMouse, and MouseOffset to offer flexibility and meet various use cases.
By following these guidelines and implementing tailored zoom modes, you can achieve smooth and effective zooming from the mouse location while maintaining the desired image position and scale factor.
The above is the detailed content of Why Does My Image Jump When Zooming from the Mouse Cursor Using C# Transformations?. For more information, please follow other related articles on the PHP Chinese website!
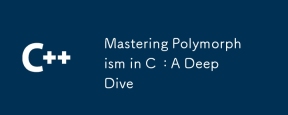
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
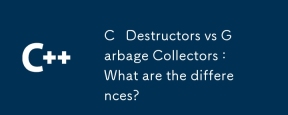
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
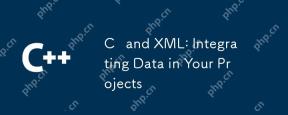
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
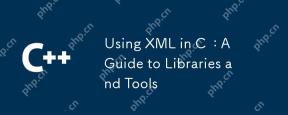
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
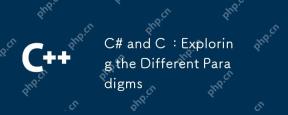
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
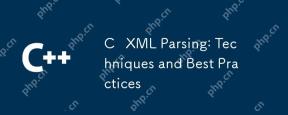
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
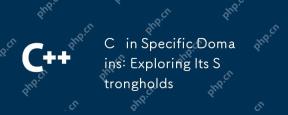
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
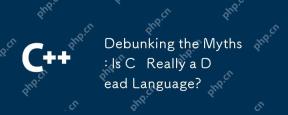
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
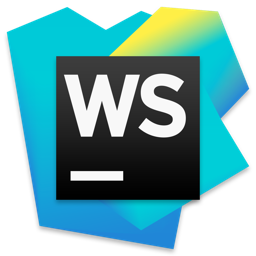
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
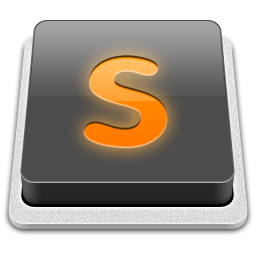
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
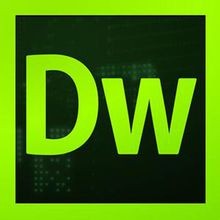
Dreamweaver CS6
Visual web development tools
