


How to Parse URI Query Strings into Name-Value Pairs in Java Without External Libraries?
Parsing URI Strings into Name-Value Collections
Introduction
Parsing URI strings into their constituent elements is a common task in web development. To accomplish this task, Java provides several built-in capabilities and external libraries. This article focuses on a Java-based solution for parsing URI strings without using external dependencies.
Parsing Query Parameters
The query portion of a URI contains a series of name-value pairs separated by the '&' character. To parse these parameters into a map, you can use the following method:
public static Map<string string> splitQuery(URL url) throws UnsupportedEncodingException { Map<string string> query_pairs = new LinkedHashMap(); String query = url.getQuery(); String[] pairs = query.split("&"); for (String pair : pairs) { int idx = pair.indexOf("="); query_pairs.put(URLDecoder.decode(pair.substring(0, idx), "UTF-8"), URLDecoder.decode(pair.substring(idx + 1), "UTF-8")); } return query_pairs; }</string></string>
Sample Usage
Using the given URL as an example:
https://google.com.ua/oauth/authorize?client_id=SS&response_type=code&scope=N_FULL&access_type=offline&redirect_uri=http://localhost/Callback
The splitQuery method will return the following map:
{client_id=SS, response_type=code, scope=N_FULL, access_type=offline, redirect_uri=http://localhost/Callback}
Encoding Considerations
The method includes URL decoding to handle any special characters present in the parameter values.
Handling Multiple Parameters with Same Key
An updated version of the method has been provided to handle scenarios where multiple parameters have the same key. This version uses a Map
Java 8 Version
A Java 8 implementation of the method is also available, which leverages lambda expressions and streams for a concise and efficient solution.
Conclusion
By using the splitQuery method, you can easily parse URI query strings into their constituent name-value pairs without relying on external libraries. This capability is essential for various web development tasks, such as extracting user input or retrieving parameters from RESTful endpoints.
The above is the detailed content of How to Parse URI Query Strings into Name-Value Pairs in Java Without External Libraries?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
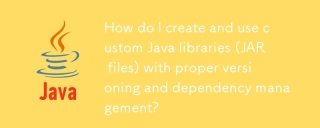
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
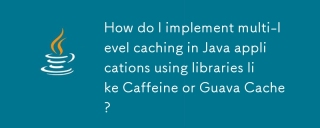
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
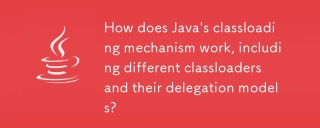
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
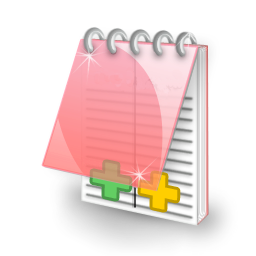
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
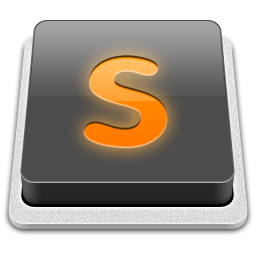
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.