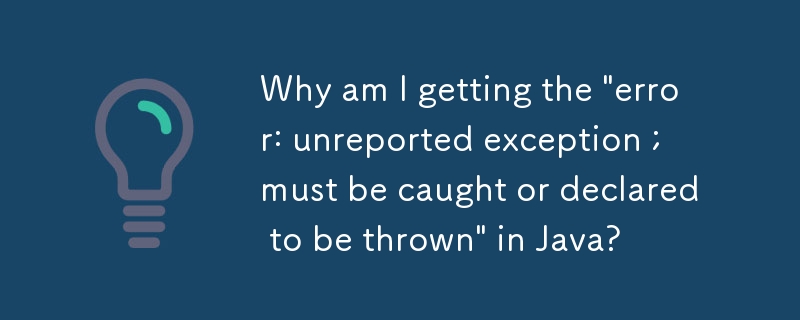
Unveiling the Enigma: "error: unreported exception" in Java
Encountering the cryptic "error: unreported exception ; must be caught or declared to be thrown" message? Don't fret; understanding this compilation error will illuminate Java concepts and guide you towards resolving it.
Unraveling the error message:
The error signifies that your code has thrown or propagated a checked exception named XXX and has failed to handle it appropriately.
Concepts behind the error:
Java classifies exceptions into two types:
-
Checked exceptions: Derived from java.lang.Throwable and Exception, excluding RuntimeException and its subclasses, these exceptions must be dealt with within their enclosing method or constructor.
-
Unchecked exceptions: Comprising Error and its subclasses and RuntimeException and its subclasses, unchecked exceptions do not need to be handled explicitly.
Resolving the issue:
To address the error, you must handle the checked exception in the code by either:
-
Catching and handling the exception: Enclosing the statement that triggers the exception within a try ... catch block, as illustrated in the code sample below:
try {
// Code that may throw the checked exception
if (someFlag) {
throw new IOException("cannot read something");
}
// Subsequent code
} catch (IOException ex) {
// Handle the exception gracefully
}
-
Declaring that the enclosing method or constructor throws the exception: By adding a throws XXX clause to the method or constructor declaration, you delegate the responsibility of handling the exception to the caller of the method or constructor. Below is an example:
public void doThings() throws IOException {
// Code that may throw an IOException
if (someFlag) {
throw new IOException("cannot read something");
}
// Subsequent code
}
Making the right choice:
The correct approach depends on the context and the desired handling of the exception within your code. Consider the level of severity and the ability to recover from the exception at the time of handling.
Special considerations:
-
Static initializers: Handling checked exceptions in static initializers requires careful handling due to syntax restrictions. Consider using static blocks instead.
-
Static blocks: Checked exceptions must be caught within static blocks because there is no enclosing context.
-
Lambdas: Lambdas cannot throw unchecked exceptions due to the function interface they use. Handle exceptions within the lambda itself.
-
Multiple exceptions: If the fully qualified names of exceptions are different despite the same name, these exceptions are distinct. Handle them individually.
Additional resources:
-
Oracle Java Tutorial:
- [The catch or specify requirement](https://docs.oracle.com/javase/tutorial/essential/exceptions/catchexceptions.html#:~:text=The catch or specify requirement)
- [Catching and handling exceptions](https://docs.oracle.com/javase/tutorial/essential/exceptions/handling.html)
- [Specifying the exceptions thrown by a method](https://docs.oracle.com/javase/tutorial/essential/exceptions/throwing.html)
The above is the detailed content of Why am I getting the 'error: unreported exception ; must be caught or declared to be thrown' in Java?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn