


How Can I Efficiently Insert Multiple Rows of Data into a Go Database Using Prepared Statements?
Efficient Multiple Data Insertion in Go
Inserting multiple data rows into a database in a single operation can significantly improve efficiency. In Go, one common way to accomplish this is through the use of prepared statements. While using string concatenation as demonstrated in your question may seem convenient, it's less secure and can lead to SQL injection vulnerabilities.
Using Prepared Statements
To insert multiple rows using prepared statements, you can follow these steps:
- Create a template SQL statement with placeholders for the data values:
INSERT INTO test(n1, n2, n3) VALUES (?, ?, ?)
- Prepare the statement using the db.Prepare method:
stmt, err := db.Prepare(sqlStr) if err != nil { // Handle error }
- Construct a slice of values for each row:
vals := []interface{}{} for _, dataRow := range data { vals = append(vals, dataRow["v1"], dataRow["v2"], dataRow["v3"]) }
- Execute the statement with the values:
res, err := stmt.Exec(vals...) if err != nil { // Handle error }
This approach ensures that the SQL statement is only parsed once and the values are securely inserted as parameters.
Example:
Here's an example of inserting multiple rows into a "test" table using prepared statements:
import ( "database/sql" "fmt" ) type DataRow struct { v1 string v2 string v3 string } func main() { data := []DataRow{ {v1: "1", v2: "1", v3: "1"}, {v1: "2", v2: "2", v3: "2"}, {v1: "3", v2: "3", v3: "3"}, } db, err := sql.Open("mysql", "user:password@tcp(localhost:3306)/database") if err != nil { // Handle error } sqlStr := "INSERT INTO test(n1, n2, n3) VALUES (?, ?, ?)" stmt, err := db.Prepare(sqlStr) if err != nil { // Handle error } vals := []interface{}{} for _, dataRow := range data { vals = append(vals, dataRow.v1, dataRow.v2, dataRow.v3) } res, err := stmt.Exec(vals...) if err != nil { // Handle error } affectedRows, err := res.RowsAffected() if err != nil { // Handle error } fmt.Printf("Inserted %d rows", affectedRows) }
The above is the detailed content of How Can I Efficiently Insert Multiple Rows of Data into a Go Database Using Prepared Statements?. For more information, please follow other related articles on the PHP Chinese website!
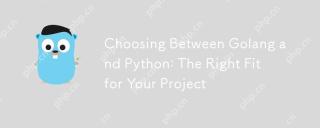
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
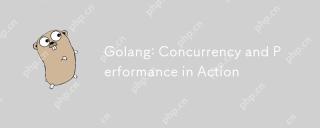
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
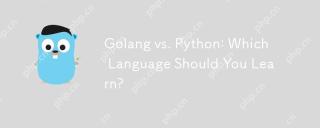
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
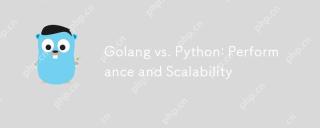
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
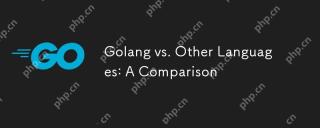
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
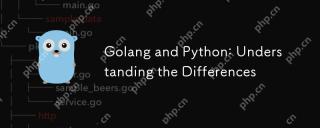
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
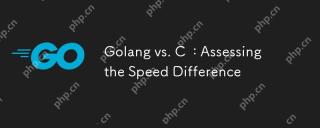
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
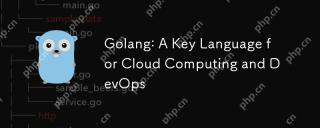
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
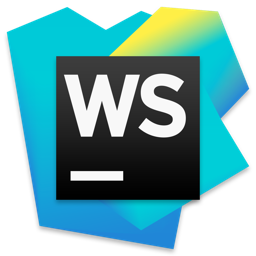
WebStorm Mac version
Useful JavaScript development tools
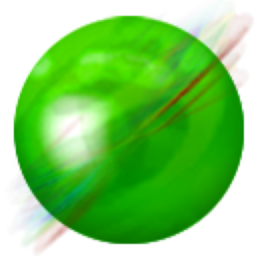
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.