


How can I repeat HTML code multiple times in a Go web application using templates?
Repeating HTML Code Multiple Times in Go
In your Go web application, you have a need to output a specific HTML line numerous times, depending on the value of n.
Here's a way to approach this:
In HTML templates, the {{range}} action allows you to iterate over items. However, this action requires a slice, array, or map to work with.
Passing a Zero-Value Slice
To cater to this requirement, you can pass an empty slice with no allocated memory, such as make([]struct{}, n).
Template Code:
-
{{range $idx, $e := .}}
- {{$idx}} {{end}}
Testing the Code:
tmpl := template.Must(template.New("").Parse(templ)) n := 5 if err := tmpl.Execute(os.Stdout, make([]struct{}, n)); err != nil { panic(err) }
Output:
Using a Filled Slice
To customize the starting index for the links, you can fill the slice with specific values.
Template Code:
-
{{range .}}
- {{.}} {{end}}
Example Test Code:
tmpl := template.Must(template.New("").Parse(templ)) n := 5 values := make([]int, n) for i := range values { values[i] = (i + 1) * 2 } if err := tmpl.Execute(os.Stdout, values); err != nil { panic(err) }
Output:
Using a Custom Function
Finally, you can use a custom function within the templates to modify the index values as needed.
Template Code:
-
{{range $idx, $e := .}}{{$idx := (Add $idx)}}
- {{$idx}} {{end}}
Custom Function:
func Add(i int) int { return i + 1 }
Output:
The above is the detailed content of How can I repeat HTML code multiple times in a Go web application using templates?. For more information, please follow other related articles on the PHP Chinese website!
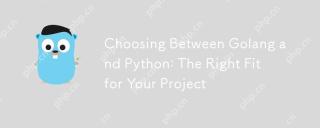
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
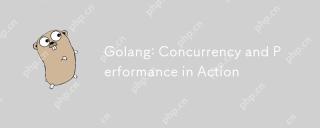
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
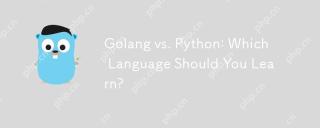
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
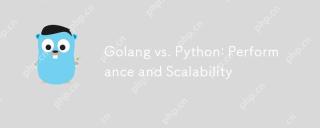
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
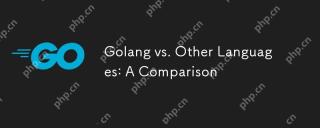
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
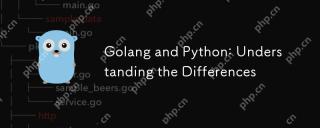
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
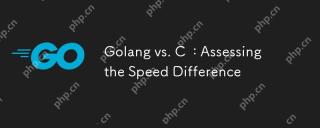
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
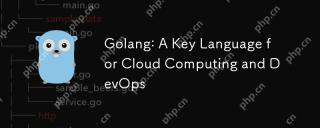
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
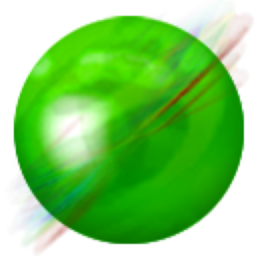
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
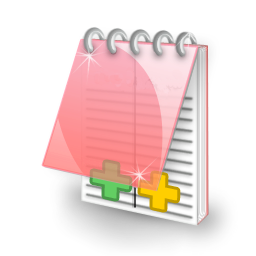
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
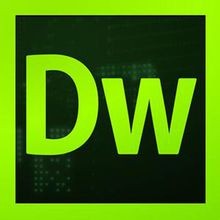
Dreamweaver CS6
Visual web development tools