


Calling Command with Some Arguments Works but Not with Others Despite Success from Console
This code snippet outputs details of 10 processes using the command /usr/bin/top with the arguments -n 10 and -l 2:
package main import ( "os/exec" ) func main() { print(top()) } func top() string { app := "/usr/bin/top" cmd := exec.Command(app, "-n 10", "-l 2") out, err := cmd.CombinedOutput() if err != nil { return err.Error() + " " + string(out) } value := string(out) return value }
However, adding the -o cpu argument (e.g., cmd := exec.Command(app, "-o cpu", "-n 10", "-l 2")) results in the error:
exit status 1 invalid argument -o: cpu /usr/bin/top usage: /usr/bin/top [-a | -d | -e | -c <mode>] [-F | -f] [-h] [-i <interval>] [-l <samples>] [-ncols <columns>] [-o <key>] [-O <secondarykey>] [-R | -r] [-S] [-s <delay>] [-n <nprocs>] [-stats <key>] [-pid <processid>] [-user <username>] [-U <username>] [-u]</username></username></processid></key></nprocs></delay></secondarykey></key></columns></samples></interval></mode>
Interestingly, the command top -o cpu -n 10 -l 2 works fine from the console in OS X 10.9.3.
The issue arises from the way arguments are separated in the Go code. The following line:
cmd := exec.Command(app, "-o cpu", "-n 10", "-l 2")
is equivalent to using the command top "-o cpu" "-n 10" "-l 2" in a shell. Most commands strictly parse arguments in this format. Therefore, top splits off the -o cpu as the first option, leaving the rest as its argument. This works for numerical arguments but fails when looking for a field named " cpu", causing the error.
To resolve this problem, separate the arguments as follows:
cmd := exec.Command(app, "-o", "cpu", "-n", "10", "-l", "2")
The above is the detailed content of Why Does My Go Code Fail to Execute the `top` Command with Certain Arguments While the Same Command Works from the Console?. For more information, please follow other related articles on the PHP Chinese website!
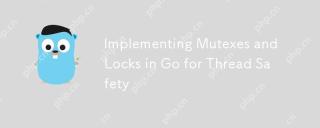
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
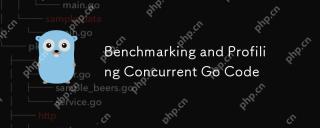
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
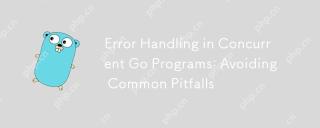
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
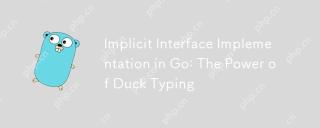
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
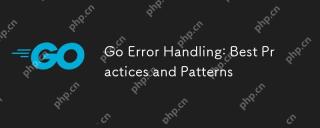
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
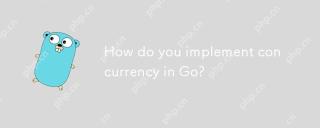
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
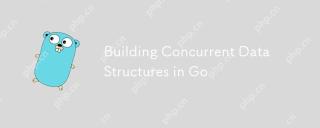
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
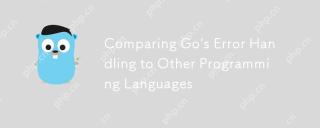
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
