URL Parameters and Query Strings in React Router v6
URL parameters and query strings are important aspects of URL management in web applications. They allow you to pass dynamic data to different routes and manage routing based on that data. React Router v6 provides seamless support for handling URL parameters and query strings, allowing you to build more dynamic and flexible applications.
1. URL Parameters in React Router v6
URL parameters, also known as route parameters or dynamic parameters, are parts of the URL that can be used to capture dynamic values. These are typically used to identify specific resources or entities.
Example of URL Parameter:
For a route like /profile/:username, the username part is a URL parameter.
Step 1: Define Route with URL Parameters
import React from 'react'; import { BrowserRouter as Router, Routes, Route, Link, useParams } from 'react-router-dom'; const Profile = () => { // Use the useParams hook to access URL parameters const { username } = useParams(); return <h2 id="Profile-of-username">Profile of {username}</h2>; }; const App = () => { return ( <router> <nav> <ul> <li> <link to="/profile/john">John's Profile</li> <li> <link to="/profile/sarah">Sarah's Profile</li> </ul> </nav> <routes> <route path="/profile/:username" element="{<Profile"></route>} /> </routes> </router> ); }; export default App;
Explanation:
- The :username in the route /profile/:username is a URL parameter.
- The useParams hook is used inside the Profile component to access the value of the URL parameter (username).
- When the user navigates to /profile/john, the value "john" will be passed as a parameter, and it will be displayed in the Profile component.
Key Takeaways for URL Parameters:
- Dynamic Route Matching: URL parameters allow you to match dynamic routes. For instance, /profile/:username can match /profile/john or /profile/sarah.
- Accessing Parameters: Use useParams to access the values of parameters in the route.
2. Query Strings in React Router v6
Query strings are key-value pairs that appear after a ? in the URL. They are typically used to pass additional information to the server or to modify the behavior of a page without changing the route.
Example of Query String:
For a URL like /search?query=React, the query string is ?query=React.
Step 1: Handling Query Strings with useLocation
In React Router v6, query strings can be accessed using the useLocation hook. useLocation provides access to the current URL, including the pathname, search (query string), and hash.
import React from 'react'; import { BrowserRouter as Router, Routes, Route, Link, useParams } from 'react-router-dom'; const Profile = () => { // Use the useParams hook to access URL parameters const { username } = useParams(); return <h2 id="Profile-of-username">Profile of {username}</h2>; }; const App = () => { return ( <router> <nav> <ul> <li> <link to="/profile/john">John's Profile</li> <li> <link to="/profile/sarah">Sarah's Profile</li> </ul> </nav> <routes> <route path="/profile/:username" element="{<Profile"></route>} /> </routes> </router> ); }; export default App;
Explanation:
- useLocation: This hook provides the current location object, which includes the pathname, search (query string), and hash.
- URLSearchParams: This is used to parse the query string and extract the values of query parameters.
- In the example, the query parameter query is extracted from the URL, and its value is displayed in the Search component.
Key Takeaways for Query Strings:
- useLocation: To access the current location, including the query string.
- URLSearchParams: A convenient API to parse and extract query parameters.
3. Combining URL Parameters and Query Strings
You can also use both URL parameters and query strings in the same route. For example, you might want to show a user profile based on a dynamic username and filter the data using query parameters.
Example with Both URL Parameters and Query Strings:
import React from 'react'; import { BrowserRouter as Router, Routes, Route, Link, useLocation } from 'react-router-dom'; const Search = () => { // Use the useLocation hook to access the query string const location = useLocation(); const queryParams = new URLSearchParams(location.search); const query = queryParams.get('query'); // Extract query parameter from the URL return ( <div> <h2 id="Search-Results">Search Results</h2> {query ? <p>Searching for: {query}</p> : <p>No search query provided</p>} </div> ); }; const App = () => { return ( <router> <nav> <ul> <li> <link to="/search?query=React">Search for React</li> <li> <link to="/search?query=JavaScript">Search for JavaScript</li> </ul> </nav> <routes> <route path="/search" element="{<Search"></route>} /> </routes> </router> ); }; export default App;
Explanation:
- In this example, the URL parameter username and the query string age are used together in the /profile/:username?age=
URL. - The username is accessed using useParams and the age filter is accessed using useLocation and URLSearchParams.
Key Takeaways:
- Combining Parameters: You can combine both URL parameters and query strings to create more complex routing scenarios.
- Separate Concerns: URL parameters are typically used for resource identification (e.g., user profile), while query strings are used for additional filtering or configuration (e.g., search query, filters).
Conclusion
React Router v6 makes it simple to handle both URL parameters and query strings in your routing logic. Using the useParams hook, you can easily access dynamic route parameters, while useLocation and URLSearchParams help manage query strings. By understanding and using these tools effectively, you can create dynamic and flexible React applications with enhanced routing capabilities.
The above is the detailed content of Mastering URL Parameters and Query Strings in React Router v6. For more information, please follow other related articles on the PHP Chinese website!
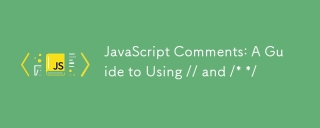
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
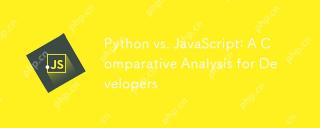
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
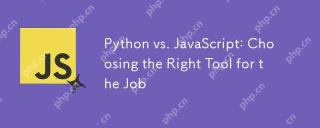
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
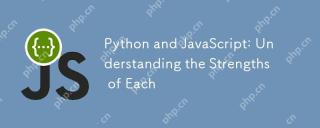
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
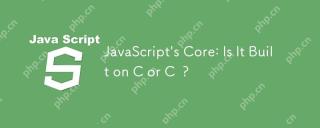
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
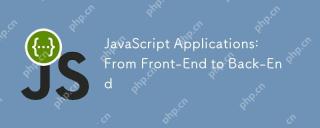
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
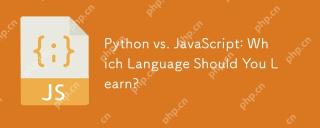
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
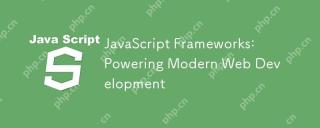
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
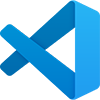
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version
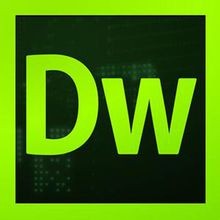
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
