


Why Doesn't Go's `json.Marshal()` Serialize Struct Fields with Lowercase Prefixes?
Exploring JSON Generation Restrictions for Struct Fields with Lowercase Prefixes in Go
In Go, developers frequently encounter an issue while attempting to generate JSON from structs that possess fields with lowercase prefix characters. Understanding the underlying reason is critical for effectively resolving this problem.
The issue arises due to Go's convention for identifying public identifiers within a package. Identifiers with an uppercase initial letter are considered public and can be accessed outside the package. Conversely, identifiers with a lowercase initial letter are deemed private and are only visible within the current package.
Consider the following code snippet:
type Machine struct { m_ip string m_type string m_serial string }
When attempting to generate JSON from an instance of this struct using json.Marshal(), you might encounter an unexpected result of just "{}." This is because the fields m_ip, m_type, and m_serial are not visible to json.Marshal() as they are considered private (indicated by the lowercase prefixes).
However, changing the fields to uppercase, as seen below, will resolve the issue:
type Machine struct { MachIp string MachType string MachSerial string }
By utilizing uppercase prefixes, you make these fields publicly accessible, allowing json.Marshal() to successfully generate the desired JSON output.
If you require lowercase identifiers in your JSON output, you can employ field tagging. By annotating each field with the corresponding JSON name, you can override the default behavior. For instance:
type Machine struct { MachIp string `json:"m_ip"` MachType string `json:"m_type"` MachSerial string `json:"m_serial"` }
Through this tagging mechanism, you can explicitly specify the desired JSON field names, regardless of their initial character case, thus enabling you to create JSON outputs that align with your application's requirements.
The above is the detailed content of Why Doesn't Go's `json.Marshal()` Serialize Struct Fields with Lowercase Prefixes?. For more information, please follow other related articles on the PHP Chinese website!
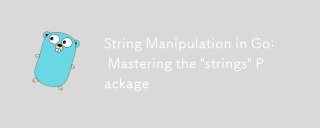
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
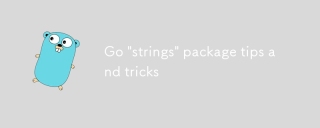
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
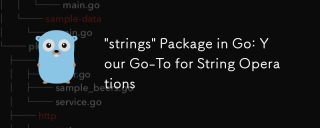
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
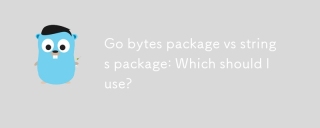
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
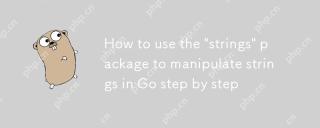
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
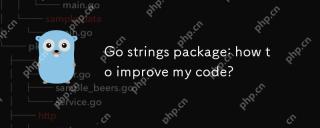
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
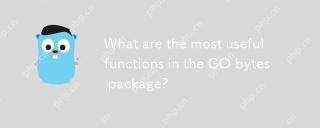
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
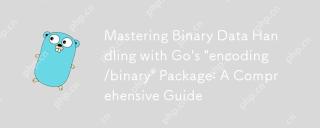
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
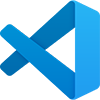
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
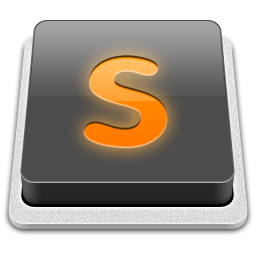
SublimeText3 Mac version
God-level code editing software (SublimeText3)
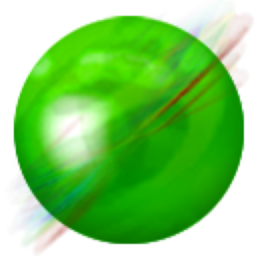
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
