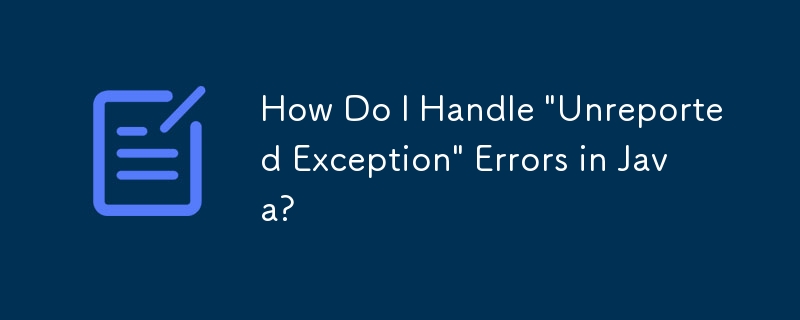
Understanding the "Unreported Exception" Error in Java
Java programmers often encounter errors like "error: unreported exception ; must be caught or declared to be thrown," where XXX is an exception class name. This error signifies that a checked exception is being thrown or propagated without being handled properly within the current scope.
Checked and Unchecked Exceptions
Java exceptions are categorized as either checked or unchecked. Checked exceptions, derived from Throwable but excluding RuntimeException and its subclasses, must be dealt with directly within the method or constructor where they occur. Unchecked exceptions, such as Error and RuntimeException and its subclasses, do not require explicit handling.
Handling Checked Exceptions
To address a checked exception, you can either:
-
Catch and Handle: Use a try ... catch statement to handle the exception within the current scope, as seen in this example:
try {
// Do some operations
if (condition) {
throw new IOException("Cannot read file");
}
// Continue operations
} catch (IOException ex) {
// Handle the exception
}
-
Declare as Thrown: Declare that the enclosing method or constructor throws the exception, passing the responsibility of handling it to the caller, as shown below:
public void doThings() throws IOException {
// Perform the same operations as in the catch-handle example
}
Deciding the Appropriate Approach
The appropriate handling method depends on the context and the nature of the exception. Consider the following guidelines:
- Handle exceptions at the level where you can effectively address them.
- Do not catch Exception or Throwable, as it can lead to catching unexpected exceptions.
- Do not declare methods as throwing Exception, as it burdens the caller with handling all potential checked exceptions.
- Avoid squashing exceptions, as it can make diagnosing runtime errors more difficult.
Special Cases
-
Static Initializers: Checked exceptions in static initializers can be handled using static blocks.
-
Static Blocks: Checked exceptions within static blocks must be caught within the block since there is no enclosing context.
-
Lambdas: Lambdas typically cannot throw unchecked exceptions, as determined by the function interface they are implementing.
-
Duplicate Exception Names: Ensure that exceptions with the same name have different fully qualified names, or the compiler will not recognize them as distinct exceptions.
Additional Resources
-
Oracle Java Tutorial:
- The catch or specify requirement
- Catching and handling exceptions
- Specifying the exceptions thrown by a method
The above is the detailed content of How Do I Handle 'Unreported Exception' Errors in Java?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn