Multi-File Upload with HTML and PHP
Enhancing the functionality of single-file uploads, HTML5 now enables the selection and submission of multiple files simultaneously. This article addresses the challenge of implementing such a feature using HTML and PHP.
In the HTML markup, the element with the type attribute set to "file" is used to create a file input control. Additionally, the multiple attribute allows users to select multiple files at once.
Within the form tag, the enctype attribute must be set to "multipart/form-data" to indicate that the form data will be sent in multiple parts. This is necessary for handling file uploads.
On the server-side, PHP uses the global $_FILES superglobal to process the uploaded files. By iterating through the array of files in $_FILES, developers can access information such as file names, temporary file locations, types, sizes, and any errors encountered during the upload process.
Below is an example script that demonstrates the process of handling multiple file uploads using HTML and PHP:
<?php if (isset($_FILES['my_files'])) { $files = $_FILES['my_files']; $fileCount = count($files["name"]); for ($i = 0; $i < $fileCount; $i++) { // File information echo "<p>File #" . ($i + 1) . ":"; echo "<p>Name: " . $files["name"][$i] . "<br>"; echo "Temporary file: " . $files["tmp_name"][$i] . "<br>"; echo "Type: " . $files["type"][$i] . "<br>"; echo "Size: " . $files["size"][$i] . "<br>"; echo "Error: " . $files["error"][$i] . "<br>"; echo "</p>"; } } ?>
By combining these techniques, developers can seamlessly enable the selection and upload of multiple files through a single file input control in web applications.
The above is the detailed content of How to Implement Multi-File Uploads Using HTML and PHP?. For more information, please follow other related articles on the PHP Chinese website!
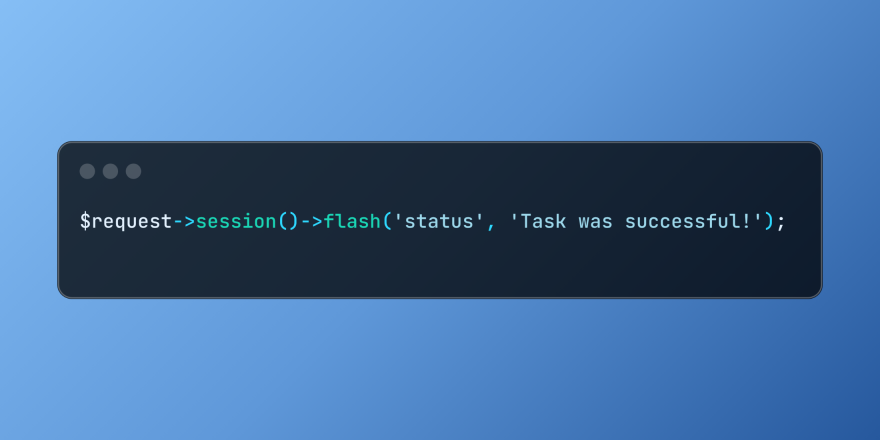
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
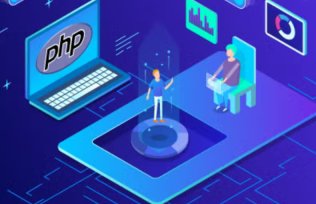
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
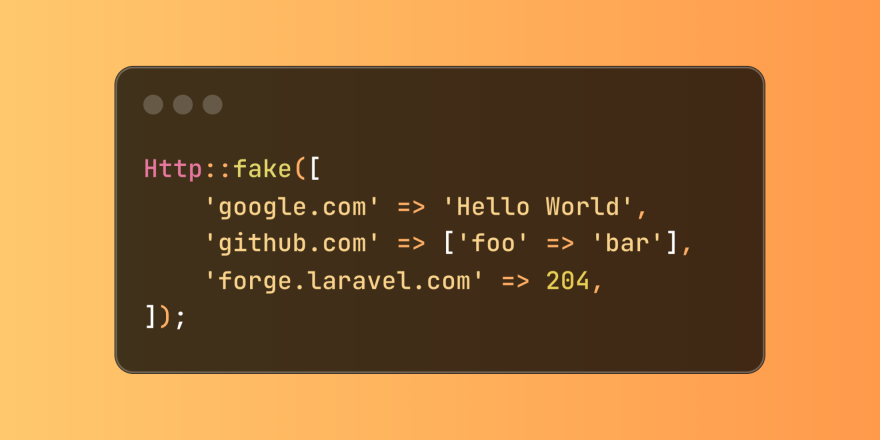
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
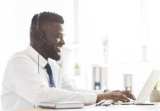
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
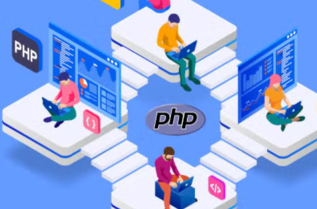
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
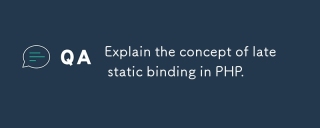
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
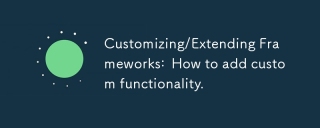
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.

Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
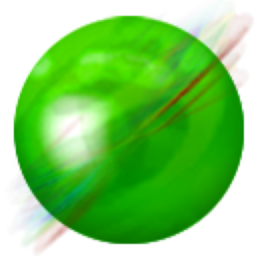
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
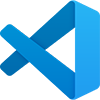
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),