


Why Does This Recursive Tax Calculation Function Result in Undefined Recursion?
Undefined Recursion in Tax Calculation
In this recursive tax calculation function:
function taxes(tax, taxWage) { var minWage = firstTier; //defined as a global variable if (taxWage > minWage) { //calculates tax recursively calling two other functions difference() and taxStep() tax = tax + difference(taxWage) * taxStep(taxWage); var newSalary = taxWage - difference(taxWage); taxes(tax, newSalary); } else { returnTax = tax + taxWage * taxStep(taxWage); return returnTax; } }
the recursion fails to terminate. Specifically, the issue lies in the arm of the function that invokes the recursive call:
if (taxWage > minWage) { // calculates tax recursively calling two other functions difference() and taxStep() tax = tax + difference(taxWage) * taxStep(taxWage); var newSalary = taxWage - difference(taxWage); taxes(tax, newSalary); }
Here, the function does not return any value or set the returnTax variable. When a function does not return explicitly, it defaults to returning undefined. Consequently, the recursion continues indefinitely, leading to undefined results.
To fix this issue, you should modify this part of the code as follows:
if (taxWage > minWage) { // calculates tax recursively calling two other functions difference() and taxStep() tax = tax + difference(taxWage) * taxStep(taxWage); var newSalary = taxWage - difference(taxWage); return taxes(tax, newSalary); }
This change ensures that the function returns the result of the recursive call, properly propagating the values up the recursion chain.
The above is the detailed content of Why Does This Recursive Tax Calculation Function Result in Undefined Recursion?. For more information, please follow other related articles on the PHP Chinese website!
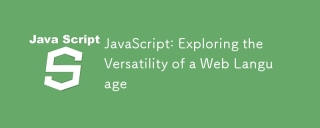
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
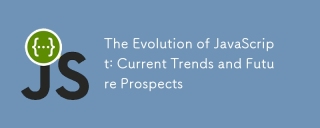
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
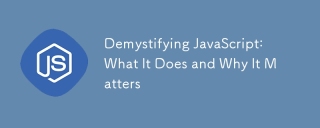
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
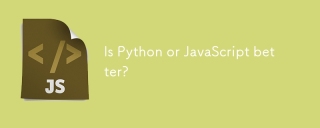
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.
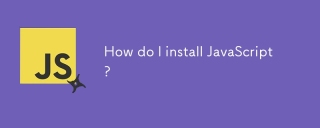
JavaScript does not require installation because it is already built into modern browsers. You just need a text editor and a browser to get started. 1) In the browser environment, run it by embedding the HTML file through tags. 2) In the Node.js environment, after downloading and installing Node.js, run the JavaScript file through the command line.
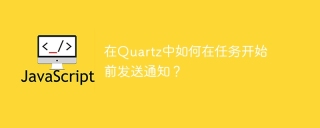
How to send task notifications in Quartz In advance When using the Quartz timer to schedule a task, the execution time of the task is set by the cron expression. Now...
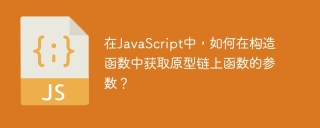
How to obtain the parameters of functions on prototype chains in JavaScript In JavaScript programming, understanding and manipulating function parameters on prototype chains is a common and important task...
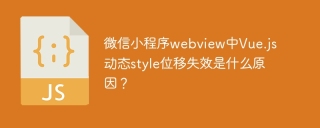
Analysis of the reason why the dynamic style displacement failure of using Vue.js in the WeChat applet web-view is using Vue.js...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor
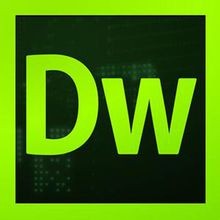
Dreamweaver CS6
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool