


Why Doesn't Appending to a Struct Slice Work in Go When Called From Another Method?
Cannot Append to Struct Slice Outside of Method
When working with slices in Go, a common issue arises when appending to a slice that is a property of a struct. In certain scenarios, this operation may fail despite working correctly when performed directly on the struct.
Consider the following example:
type Test1 struct { all []int } func (c Test1) run() []int { for i := 0; i <p>Here, the code successfully appends to the all slice within the run method of the Test1 struct. However, if this method calls another method, the append operation fails.</p><p>For example:</p><pre class="brush:php;toolbar:false">type Test3 struct { all []int } func (c Test3) run() []int { c.combo() return c.all } func (c Test3) combo() { for i := 0; i <p>In this scenario, the Test3.combo method attempts to append to the all slice but fails. The reason for this is that Go passes values by value, meaning a copy of the Test3 struct is created when c.combo() is called.</p><p>The copy operates on its own slice, and when the method returns, the associated changes are discarded. Therefore, when Test3.run tries to return c.all, it returns an empty slice because the copy's modified version is lost.</p><p>The solution to this issue is to use a pointer receiver for the combo method:</p><pre class="brush:php;toolbar:false">func (c *Test3) combo() { for i := 0; i <p>Using a pointer receiver ensures that the method operates on the original Test3 struct, allowing changes made within the combo method to be preserved when it returns.</p>
The above is the detailed content of Why Doesn't Appending to a Struct Slice Work in Go When Called From Another Method?. For more information, please follow other related articles on the PHP Chinese website!
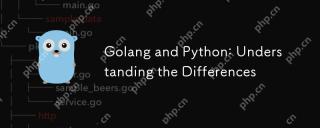
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
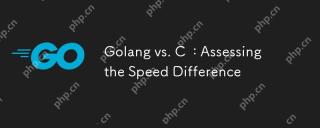
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
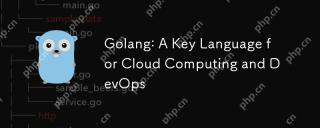
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
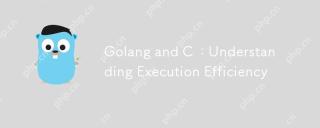
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
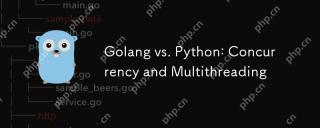
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
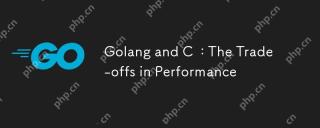
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
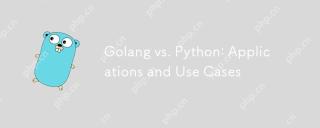
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool