How to Efficiently Save PNG Images Server-Side from a Base64 Data URI
When creating images on the client side using a tool like Canvas2Image, there often arises a need to convert the resulting base64 strings into actual PNG files on the server. This can be achieved effectively using PHP's base64_decode() function.
Extracting and Decoding the Base64 Data
To extract the image data from the base64 string, follow these steps:
$data = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAABE...'; list($type, $data) = explode(';', $data); list(, $data) = explode(',', $data); $data = base64_decode($data);
This code line-by-line:
- Splits the string using ; to separate the data type and the actual data.
- Ignores the first element, which is the data type, and keeps only the actual data.
- Decodes the base64-encoded data into its binary form using base64_decode().
Saving the PNG File
Once the data is extracted and decoded, you can simply save it to the server as a PNG file using file_put_contents():
file_put_contents('/tmp/image.png', $data);
One-Liner Solution:
Alternatively, you can combine the extraction, decoding, and saving into a concise one-liner:
$data = base64_decode(preg_replace('#^data:image/\w+;base64,#i', '', $data));
Error Handling:
To ensure data integrity, consider validating the type of image and checking for potential errors during base64 decoding:
if (preg_match('/^data:image\/(\w+);base64,/', $data, $type)) { // ... (additional error handling and processing) }
The above is the detailed content of How to Efficiently Save Server-Side PNG Images from a Base64 Data URI?. For more information, please follow other related articles on the PHP Chinese website!
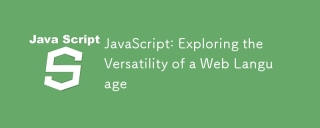
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
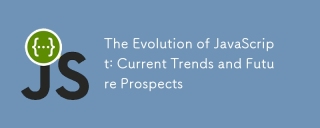
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
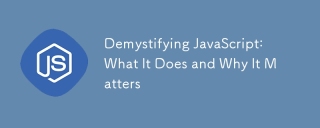
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
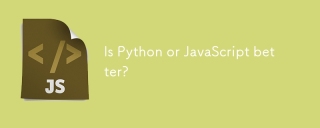
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.
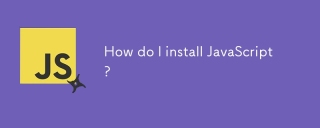
JavaScript does not require installation because it is already built into modern browsers. You just need a text editor and a browser to get started. 1) In the browser environment, run it by embedding the HTML file through tags. 2) In the Node.js environment, after downloading and installing Node.js, run the JavaScript file through the command line.
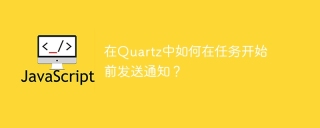
How to send task notifications in Quartz In advance When using the Quartz timer to schedule a task, the execution time of the task is set by the cron expression. Now...
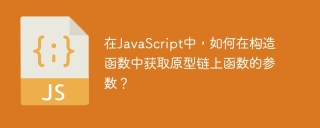
How to obtain the parameters of functions on prototype chains in JavaScript In JavaScript programming, understanding and manipulating function parameters on prototype chains is a common and important task...
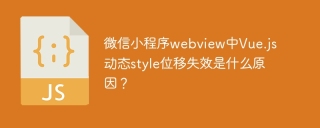
Analysis of the reason why the dynamic style displacement failure of using Vue.js in the WeChat applet web-view is using Vue.js...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
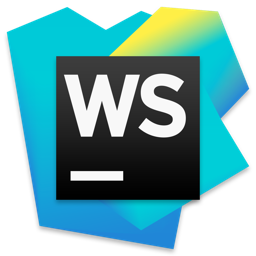
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.