Binding Member Functions to Generic std::function Objects
In C , you may encounter situations where you need to store function pointers to member functions of a particular class in a container that accepts std::function objects. However, attempting to bind a non-static member function directly to a std::function can lead to errors.
Consider the following code:
#include <functional> class Foo { public: void doSomething() {} void bindFunction() { // ERROR std::function<void> f = &Foo::doSomething; } };</void></functional>
This code will result in a compiler error because non-static member functions implicitly accept a "this" pointer as their first argument. To resolve this issue, you need to bind the object in advance using the std::bind function.
std::function<void> f = std::bind(&Foo::doSomething, this);</void>
This version correctly binds the "this" pointer to the member function, allowing you to store it in the std::function object without triggering the error.
For member functions that accept parameters, you can use std::placeholders to specify their positions:
using namespace std::placeholders; std::function<void> f = std::bind(&Foo::doSomethingArgs, this, _1, _2);</void>
Alternatively, if your compiler supports C 11 lambdas, you can use them to create the std::function object:
std::function<void> f = [=](int a, int b) { this->doSomethingArgs(a, b); }</void>
By utilizing these techniques, you can effectively bind member functions to generic std::function objects, enabling you to store and manipulate them conveniently.
The above is the detailed content of How to Correctly Bind C Member Functions to std::function Objects?. For more information, please follow other related articles on the PHP Chinese website!
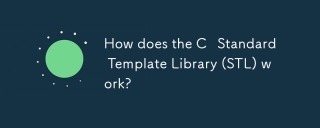
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
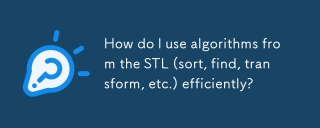
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
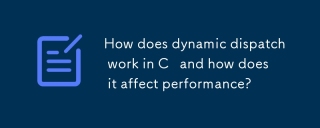
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
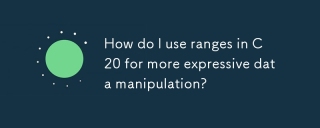
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
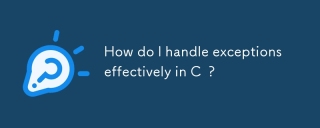
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
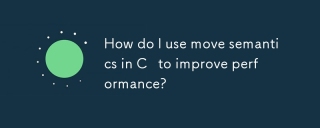
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
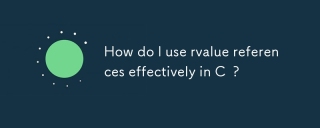
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
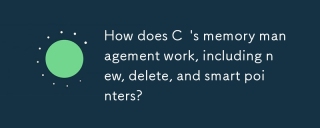
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
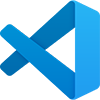
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
