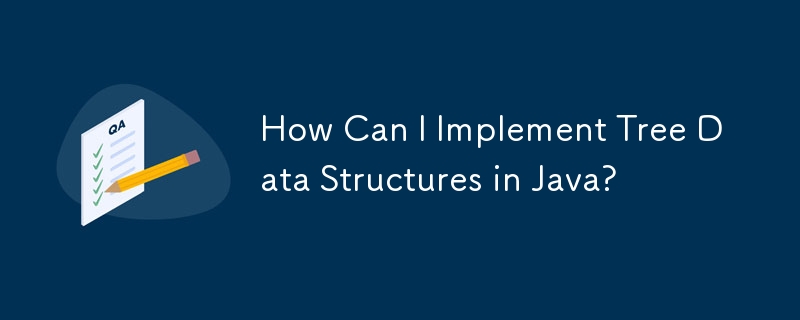
Implementing Tree Data Structures in Java
When working with complex data organizations, tree data structures offer a powerful solution. Java provides various options for representing trees, ensuring flexibility for specific requirements.
Standard Java Library for Trees
Regrettably, the Java Standard Library lacks a dedicated tree data structure. However, you may consider using existing data structures, such as:
-
HashMap: By exploiting keys and values, a HashMap can simulate a tree with a single child per node.
-
LinkedHashSet: This data structure supports ordered insertion and can mimic a tree with multiple children per node.
Custom Tree Implementation
If these options don't meet your needs, it's advisable to create a custom tree implementation. The provided Python example demonstrates a basic tree structure:
class Tree:
def __init__(self, root_data):
self.root = Node(root_data)
class Node:
def __init__(self, data):
self.data = data
self.children = []
This implementation allows:
-
Unlimited Children for Each Node: The Node class can have multiple children.
-
String Values for Nodes: Both the root and child nodes can hold string values.
-
Retrieving Children and Values: A method can be added to the Node class to retrieve all child nodes and their string values for efficient access.
Additional Considerations
-
Traversal: Implement methods for pre-order, in-order, and post-order traversal to navigate the tree efficiently.
-
Adding and Removing Nodes: Define methods to add and remove nodes while maintaining the tree structure.
-
Serialization and Deserialization: Consider serializing/deserializing the tree to support storage and retrieval from external sources.
The above is the detailed content of How Can I Implement Tree Data Structures in Java?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn