


Automating Debian Package Update Summaries with Python and Gemini (gemini--flash)
If you are using a Debian-like distro and are a new user or just starting your career as a sysadmin, you probably already know the importance of updating packages using apt update. You might also want to understand what each package does to learn more about Linux. Additionally, system administrators (sysadmins) often need to communicate or document to stakeholders which updates are urgent or security-related.
In this post, I will show you how to combine Python, the apt list -u command, and Gemini AI to create human-readable summaries of pending package updates.
The Goal ?
- Retrieve the list of pending updates on Debian using the apt list -u command. Note: If desired, you can modify the output using something like:
apt list -u | awk '{ print }' | sed 's|/.*||'
- Send this list to Gemini AI (using Google's generative library).
- Use the AI to categorize and summarize the importance of each package update.
- Save the results in a Markdown file to facilitate sharing.
Requirements ?
- Python 3.8
- Google Gemini API Key
- Required Libraries:pip install google-generativeai environs
- Debian-Based System: This script relies on the apt command.
The Code
Here’s a breakdown of the solution across two scripts:
apt_list.py
This script runs apt list -u to fetch pending updates, processes the output, and uses the prompt function to get categorized summaries from Gemini AI.
import subprocess from utils.gemini_cfg import prompt try: # Run 'apt list -u' to list upgradable packages result = subprocess.run(["apt", "list", "-u"], capture_output=True, text=True, check=True) output = result.stdout # Get command output # Use the Gemini AI model to summarize the updates summary = prompt(output) # Save the AI-generated summary to a Markdown file with open("./gemini_result.md", "w") as file: file.write(summary) print("Summary saved to gemini_result.md") except subprocess.CalledProcessError as e: print("Error while running apt list:", e)
gemini_cfg.py
This script configures the Gemini API and defines the prompt function for AI-generated content.
import google.generativeai as genai from environs import Env # Load API key from .env file env = Env() env.read_env() key = env("TOKEN") # Replace with your environment variable key name # Configure Gemini API genai.configure(api_key=key) model = genai.GenerativeModel("gemini-1.5-flash") # Function to prompt Gemini AI for summaries def prompt(content): message = ( "You work as a sysadmin (Debian server infrastructure). " "You must create a list categorizing the importance in terms of security and priority, " "providing a brief summary for each package so that business managers can understand " "what each library is from this output of the `apt list -u` command: " f"{content}" ) response = model.generate_content([message]) return response.text
- Run the apt_list.py script: python apt_list.py
-
The script does the following:
- Retrieves the pending Debian package updates.
- Passes the list to Gemini AI for categorization and explanation.
- Saves the AI-generated output to gemini_result.md.
Open gemini_result.md to see a clear, categorized summary of updates for easy communication.
Example Output
Here's an example of what the generated summary might look like:
## Debian Package Update List: Priority and Security The list below categorizes the packages available for update, considering their importance in terms of security and business operation priority. The classification is subjective and may vary depending on your company's specific context. **Category 1: High Priority - Critical Security (update immediately)** - **linux-generic, linux-headers-generic:** Critical kernel updates to fix security vulnerabilities. - **libcurl4:** Resolves potential security issues for data transfer operations. ... **Category 2: High Priority - Maintenance and Stability (update soon)** * **`e2fsprogs`, `logsave`:** Packages related to ext2/ext3/ext4 file systems. Update to ensure data integrity and file system stability. **Medium-High priority.** ... **Category 3: Medium Priority - Applications (update as needed)** * **`code`:** Visual Studio Code editor. Update for new features and bug fixes, but not critical for system security. * **`firefox`, `firefox-locale-en`, `firefox-locale-pt`:** Firefox browser. Updates for security fixes and new functionalities. Priority depends on Firefox usage in your infrastructure. ...
Conclusion
With a bit of Python and Gemini AI, you can automate and improve how you communicate Debian package updates. This script is an excellent foundation for integrating AI into sysadmin workflows. This post is for educational purposes, so be mindful of Gemini API resources, as well as the secure handling of your system.
Thanks for reading! ?
The above is the detailed content of Automating Debian Package Update Summaries with Python and Gemini (gemini--flash). For more information, please follow other related articles on the PHP Chinese website!
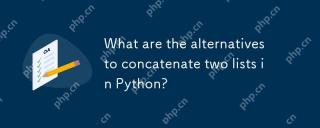
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
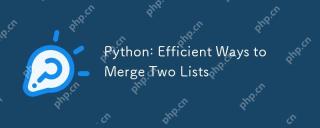
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
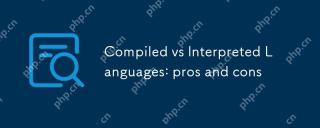
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
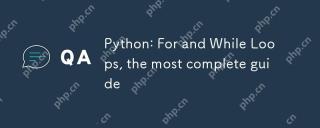
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
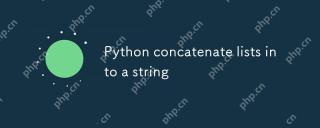
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
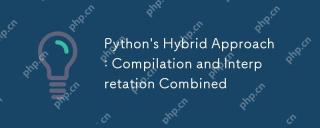
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
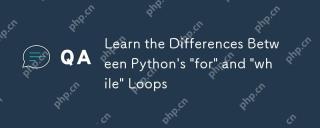
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
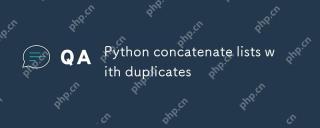
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
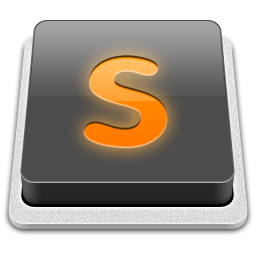
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
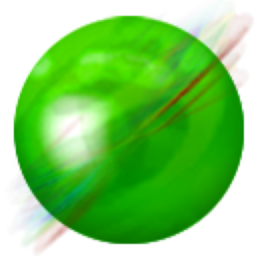
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
